This code implements insertion sort algorithm to arrange numbers of an array in ascending order. The insertion sort iterates through each element in the. The array is virtually split into a sorted and an unsorted part.
C Program to implement Insertion Sort Coding Guide for
With a little modification, it will arrange numbers in descending order.
To sort an array of size n in ascending order:
Values from the unsorted part are picked and placed at the correct position in the sorted part. Of elements in the list:\n); An insertion sort is a sorting technique used in c programming to sort elements of an array in ascending or descending order. Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands.
After receiving the inputs, sort the given array in ascending order using insertion sort as.
Insertion sort is a sorting algorithm that places an unsorted element at its suitable place in each iteration. Insertion sort works similarly as we sort cards in our hand in a card game. I++) { scanf (%d, &a[i]); Now we will pick each number from the unsorted section and insert that number at a.
An array of data, and the total number in the array.
In this tutorial, you will learn about insertion sort algorithm and its implementation in c, c++, java and python. To sort an array using insertion sort technique in c++ programming, you have to ask from user to enter the size for an array and then enter elements of that size in random order. This process will continue until array gets sorted. #include<stdio.h> int main() { int a[50], i,j,n,t;
The same approach is applied in insertion sort.
When a new element is to be inserted into the sorted list, it is compared with the elements in the sorted list and inserted at its correct place. We select one element from the unsorted set at a time and insert it into its correct position in the sorted set. Although it is simple to use, it is not appropriate for large data sets as the time complexity of insertion sort in the average case and worst case is o(n 2), where n is the number of items. In this sorting technique, we assume that the first number in the array is in the sorted section and the rest of all the other numbers in the array are in the unordered section.
Given an array of n integers, write a program to implement the insertion sorting algorithm.
Insertion sort algorithm picks elements one by one and places it to the right position where it belongs in the sorted list of elements. This insertion sort in c program allows the user to enter the array size and the one dimensional array row elements. Next, we are using nested for loop to sort the array elements using insertion sort. In this guide, we will show you how to implement the insertion sort as a separate function that takes a reference to the std::vector object and modifies the contents in place.
In insertion sort we pick first element and put it at its actual position by comparison with its right elements.
Selection sort using recursion in c. Introduction to insertion sort in c++. You should test each algorithm on random numbers consisting of lists of sizes. It will keep working on single elements and eventually put them in the right position, eventually ending with a sorted array.
Insertion sort works by picking one element at a time and places it accordingly in the array.
Introduction to insertion sort in c. Insertion sort using recursion in c. Lets see how its works The idea behind the insertion sort is that first take one element, iterate it through the sorted array.
Implement the insertion sort for the std::vector container in c++.
This is done by shifting elements which are greater than the element one position to the right. Printf (\n please enter the array elements : Best case complexity of insertion sort is o (n), average and the worst case complexity is o (n 2 ). And then select next element of an array and try to place it at its actual place after comparison.
The basic idea of this method is to place an unsorted element into its correct position in a growing sorted list of elements.
Insertion sort | logical programming in c | by mr.srinivas** for online training registration: C program for insertion sort to sort numbers. #include <stdio.h> int main () { int a [100], number, i, j, temp; Insertion sort is a simple sorting algorithm that works similar to the way you sort playing cards in your hands.
The comparison is done backwards from the last element.
Some characteristics of insertion sort in c++. After you have coded your algorithms, you need to test them, to see how much time is needed for each sorting algorithm takes to execute. Insertion sort is a sorting algorithm that helps in sorting objects of an array one by one. Insertion sort is less efficient than the other sorting.
Insertion sort is very easy and basic sorting technique.
Following is the c program to sort the elements by using the insertion sort technique −. Void insertionsort (int arr [], int n) {. We assume that the first card is already sorted then, we select an unsorted card. This article will demonstrate how to implement an insertion sort algorithm in c++.
Before going through the program, lets see the.
Write and compile a c program that will do the following: In the following c program we have implemented the same logic.
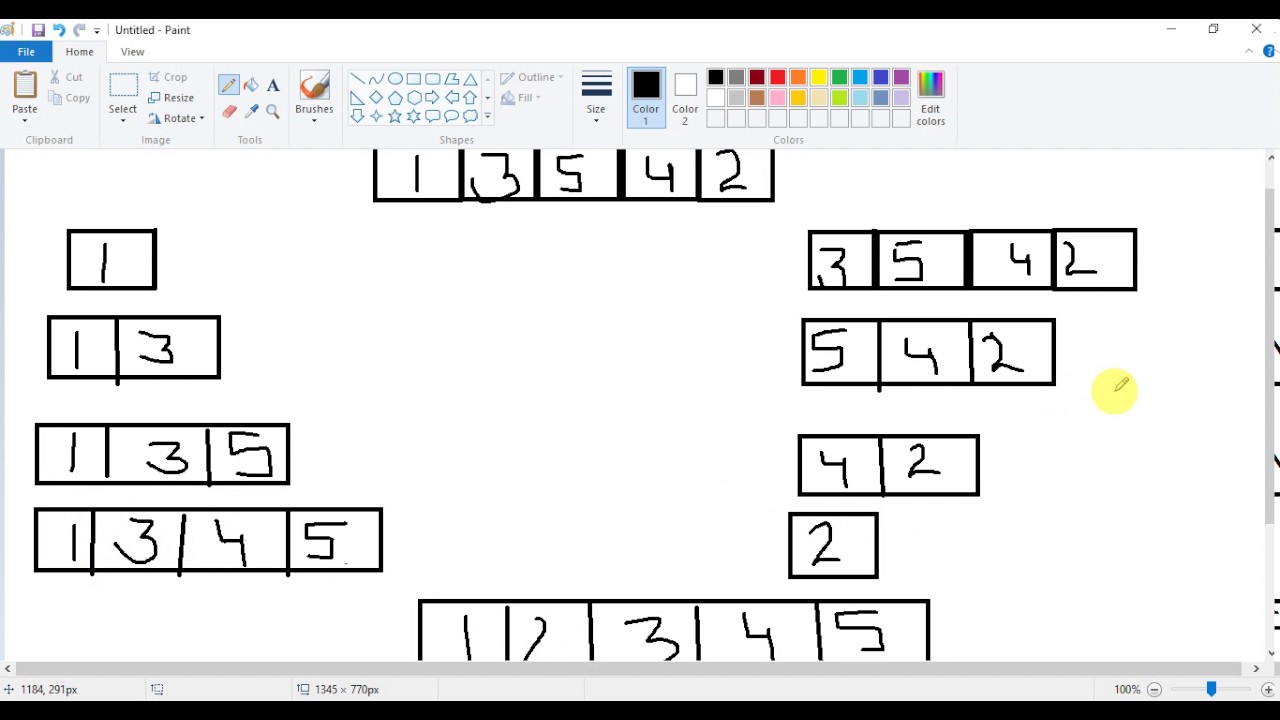


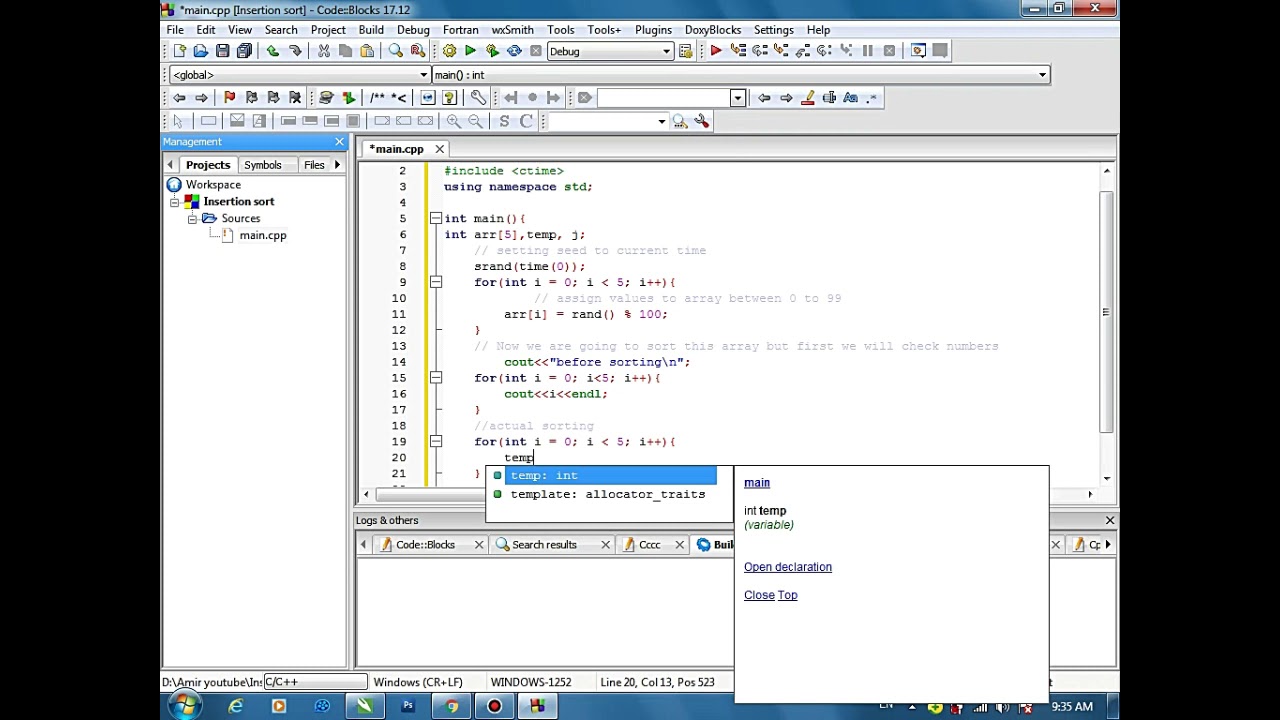


