Insertion sort's time and space complexity: So now, if the list has a length of 4, this right here will produce [1,2,3]. For j in range (1, len (arr)):
Python, Sorting Algorithm Part2. Quick Sort and Merge
} } private static void swap (comparable [] a, int i, int j) { comparable t =.
It is assumed that the first card is already sorted in the card game, and then we select an unsorted card.
In the same way, other unsorted cards are taken and put in their right place. In this article, we will learn about the implementation of insertion sort in python 3.x. Below is simple insertion sort algorithm for linked list. For i in range(1, len(arr)):
While j >=0 and key < arr [j] :
I++) { for (int j = i; In the same way, other unsorted cards are taken and put in their right place. I learned the insertion sort in ascending order. The sorted cards would appear in the left of your hand.
If the unsorted card is greater than the card in hand, it is placed on the right otherwise, to the left.
Public class sorting { public static void sort (comparable [] a) { int n = a.length; It will repeatedly happen until the all element is inserted at correct place. Insertion sort is simple sorting algorithm. For i in range (0, num_of_elements):
Insertion sort takes a similar method.
# implemntation of insertion sort in python # cards in the right hand are unsorted # cards in the left hand are sorted # initially there is only one card in left hand and remaining cards are in the right hand # function for insertion sort: Insertion sort works similarly as we sort cards in our hand in a card game. What is insertion sort in python? If the element in the sorted list is smaller than the current element, iterate to the next element.
For example, the sort of cards in the game is also insertion sort.
The element at position (j) that we need to put in the right place goes from wherever (i) is and goes down to the left all the way to its correct position; To use the insertion sort, you create two lists: Worst, best, average case time complexity and space complexity. If the selected unsorted card is greater than the first card, it will be placed at the right side;
Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands.
So, 1 would be the second to the leftmost element. Range can be invoked with three arguments start, stop and step. 3) change head of given linked list to. You aren't sorting an array.
For (int i = 1;
# pick up the first. The python insertion sort works like sorting game cards. A) insert current node in sorted way in sorted or result list. And the leftmost element is the 0th item, so we can start at the first item.
Otherwise, shift all the greater element in the list by one position towards the.
Similarly, other unsorted cards are taken and placed in their proper order. Here is a cool video on youtube demonstrating insertion sort visually in romanian folk dance Stack exchange network stack exchange network consists of 179 q&a communities including stack overflow , the largest, most trusted online community for developers to learn, share. Learn how to implement an insertion sort sorting algorithm using python.
We assume the first card to sorted, then take an unsorted card and check whether it is greater or lesser than the first card place it to the right or left to the first.
Compare 79 with the elements that are on the left. You can do this using range directly. Compare the current element with all elements in the sorted list. # temp stores current element whose left side is traversed # to find correct position temp = list_vals [i];
We choose an unsorted card since we think the first card is already sorted.
Otherwise, it goes to the left. A sorted and unsorted list. Key = 1 arr_left = [:key] = [73] key_element = x[key] = 79. It simply pick up the item from right side and place them on left side according to.
Insertion sort is a popular shorting algorithm, similar to selection sort the unsorted list or array is divided into to two parts, left part being sorted which is initially empty and the right part being unsorted which at the very beginning is the entire list.
At the second to the leftmost element. This allows you to construct a range 10, 9,.: This algorithms simply divides the list into two parts ie sorted (left side) and unsorted (right side). If the unsorted card is larger than the one in hand, it goes to the right;
This is achieved by shifting all the elements towards the right, which are larger than the current element, in the sorted array to one position ahead.
If the unsorted card is greater than the card in hand, it is placed on the right otherwise, to the left. It works in the same way as we (teachers) arranges answer sheets of students according to their roll number. 3 would be the last one. Insertion sort operates in a similar way to how we sort cards in a card game.
1) create an empty sorted (or result) list 2) traverse the given list, do following for every node.
Insertion sort is a sorting algorithm that is used to build a sorted list by placing an unsorted element at its suitable place through each iteration. Insertion sort works similarly as we sort cards in our hands in a card game. For i in range(1, len(lst)): If the current number is lesser than the number on left than swap values if alist[current] < alist[i]:
Current = i+1 while current :
2 would be the next one to the right. At each iteration element from the unsorted list is inserted at the appropriate position in the sorted part. Now, i need to do it in descending order but i have to start sorting the array from right to left. Insertion sort works similar to the sorting of playing cards in hands.
We do that by swapping ;
I've implemented my own insertion sort algorithm, and here's the code. Otherwise, it will be placed at the left side. To sort the array using insertion sort below is the algorithm of insertion sort. # for each card in right hand:
We assume that the first card is already sorted then, we select an unsorted card.
We assume that the first card is already sorted then, we select an unsorted card. # checks whether left side elements are less than current element. The unsorted cards would appear on the right until you sort them all.
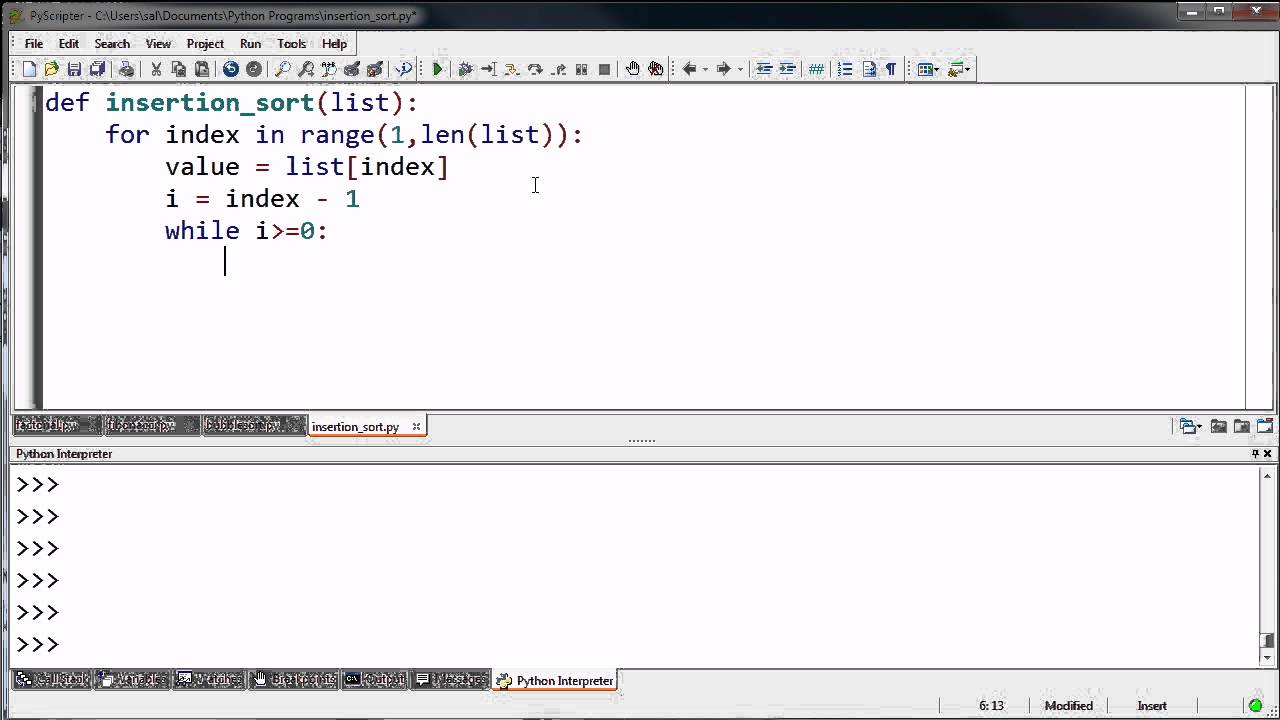


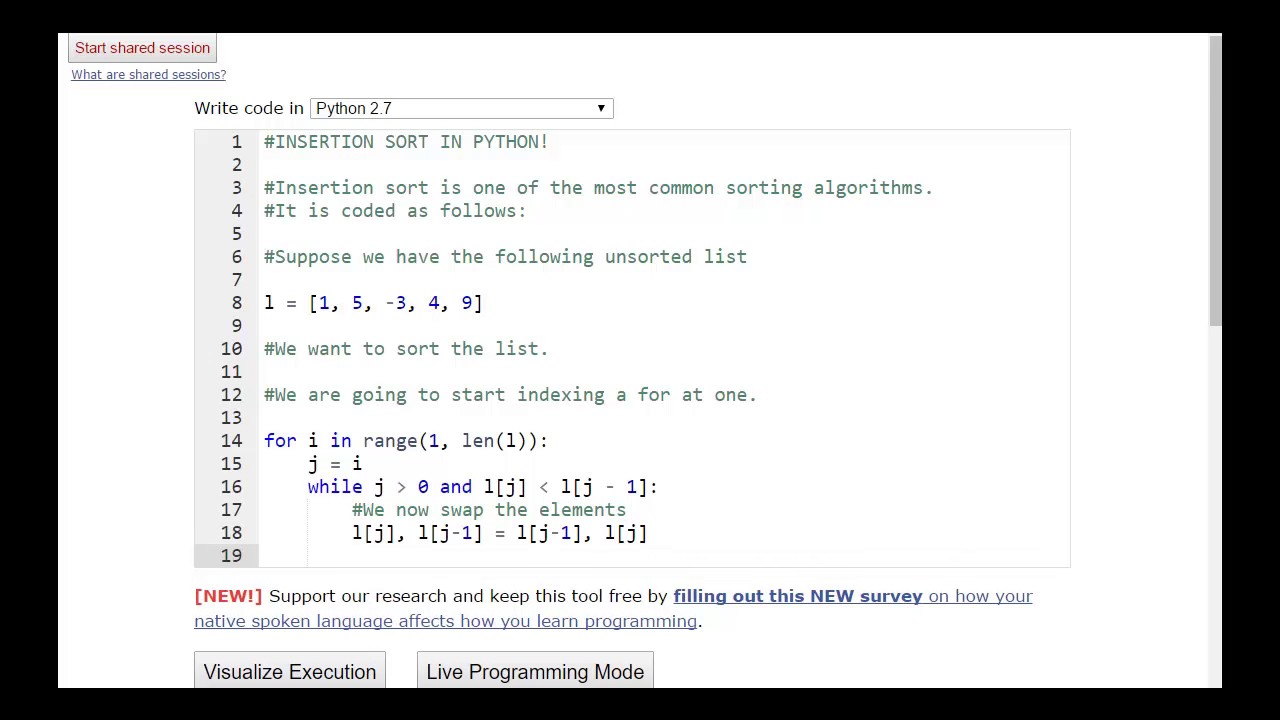


