Implementation # creating a function for insertion def insertion_sort (list1): Here is a really quick and short solution to the insertion sort algorithm in python. Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands.
Insertion Sort Algorithm Explained (Full Code Included
The array elements are compared with each other sequentially and then arranged simultaneously in some particular order.
If you haven’t read that, you can find it here.
Insertion sorting the algorithm starts at element 0 in an array and considers element sorted. We assume that the first card is already sorted then, we select an unsorted card. Hence, the process is to think of the first element as a list and realize it is ordered. The python insertion sort works like sorting game cards.
In insertion sort, you compare the key element with the previous elements.
If new > lst [chk_loc]: Insertion sort is a simple sorting algorithm for a small number of elements. This video is a part of a full algorithm series: While j >=0 and key < arr [j] :
Insertion sort is the sorting mechanism where the sorted array is built having one item at a time.
If the previous elements are greater than the key element, then you move the previous element to the next position. Photo by trevor vannoy on unsplash. Step 1 − if it is the first element, it is already sorted. To order a list of elements in ascending order, the insertion sort algorithm requires the following operations:
Values from the unsorted part are picked and placed at the correct position in the sorted part.
An index pointing at the current element indicates the position of the sort. The goal is to get an. The insertion sort algorithm works off the concept of dividing the array into two parts. We are in the second tutorial of the sorting series.
Our sorted array now has 2.
A sorted and unsorted list. An array is partitioned into a sorted subarray and an unsorted subarray. It takes place in the original list that is to be sorted. We will be using python 3.8.10.
Insertion sort is a sorting algorithm that places an unsorted element at its suitable place in each iteration.
The analogy can be understood from the style we arrange a deck of cards. In this tutorial, you will learn about insertion sort algorithm and its implementation in c, c++, java and python. Like all sorting algorithms, we consider a list to be sorted only if it is in the ascending order. Then insert one element at the time.
At the beginning of the sort (index=0), the current value is compared to the adjacent value to the left.
To use the insertion sort, you create two lists: The array is virtually split into a sorted and an unsorted part. Prv and nxt, stands for previous and next and stop, when value of nxt is greater than value of node we want ot insert. Classical way to do it is to use two pointers:
# outer loop to traverse through 1 to len (list1) for i in range (1, len (list1)):
The insertion sort algorithm takes each element of an unsorted list and, one at a time, uses comparison to insert each element into its proper place in a new, sorted list: You compare each item in the unsorted list until you sort that item. The first element in the unsorted array is evaluated so that we can insert it into its proper place in the sorted subarray. For i in range(1, len(arr)):
After looking at how the insertion sort algorithm works, we want to implement it in python.
Start from index 1 to size of the input array. Insertion sort is a simple sorting algorithm that works similar to the way you sort playing cards in your hands. Insertion sort works similarly as we sort cards in our hand in a card game. It then looks at the first element to the right of our sorted array that just contains our 0 position element it then inserts this unsorted element in to it’s correct location.
Then we insert this element into correct place, doing curr.next = next, prv.next = curr.
Insertion sort algorithm involves the sorted list created based on an iterative comparison of each element in the list with its adjacent element. Have you ever sorted playing cards in your hand? The insertion sort is a common standard algorithm in the python language. The previous tutorial talks about bubble sort which is a very simple sorting algorithm.
For each element inserted, swap it down until it is on place.
At the beginning, the sorted subarray contains only the first element of our original array. This sort works on the principle of inserting an element at a particular position, hence. To sort an array of size n in ascending order:





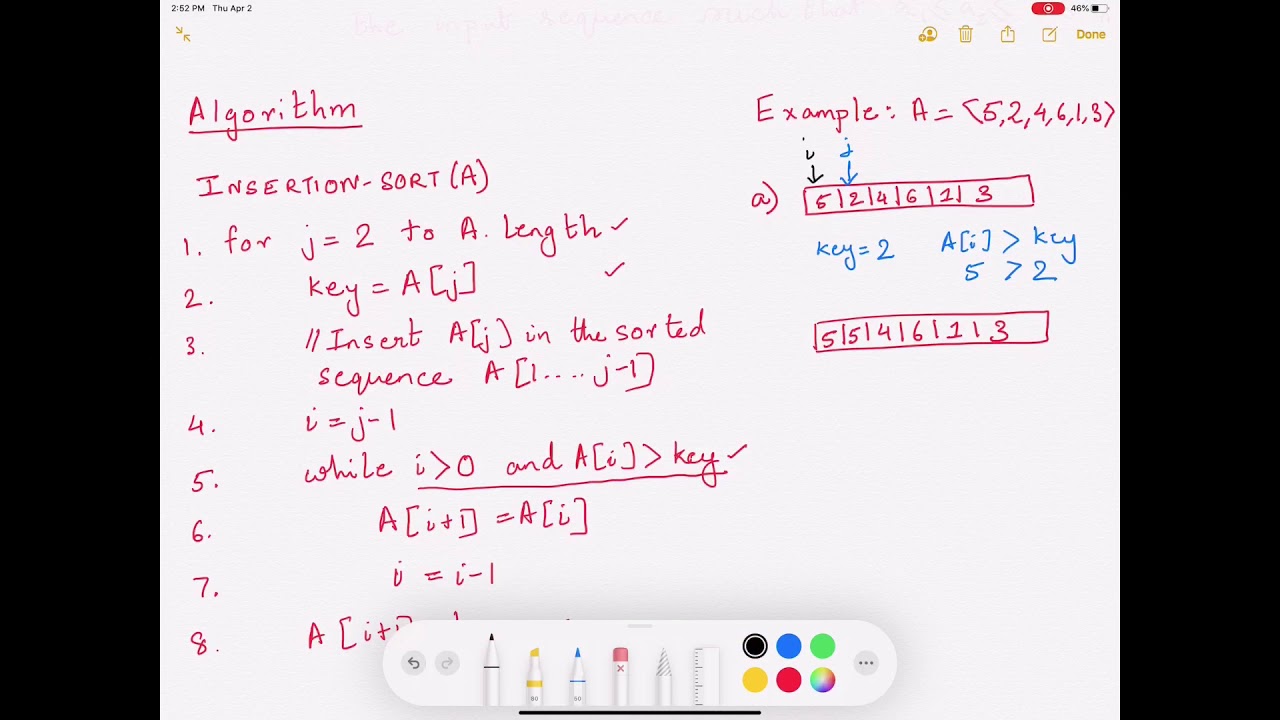