Insertion sorting is an algorithm that completes a sort by finding and inserting its position by comparing all elements of the data array with the parts of the array that are already sorted, in order from the beginning. However, insertion sort provides several advantages: Key ← a [j] 3.
Insertion Sort Algorithm Easy Explanation (With Example
Although conceptually we are sorting a sequence, then input comes to us in the form of an array with n elements.
} // function to sort an array a [] of size 'n'.
I got this pseudocode when studying schaum's outline of principles of computer science chapter 2: The analogy can be understood from the style we arrange a deck of cards. // insertion sort in c #include <stdio.h> // function to print an array void printarray(int array[], int size) { for (int i = 0; Insertion sort is a simple sorting algorithm that works similar to the way you sort playing cards in your hands.
In this post i talk about writing this in ocaml.
It’s more efficient with the partially sorted array or list, and worst with the descending order array and list. In this video i go through the basic sorting algorithm, insertion sort. The pseudocode for insertion sort of an array a is on p.18 of the book. The array is virtually split into a sorted and an unsorted part.
If you have any suggestions for future videos, please email me at keithglearning@gmai.
It builds on the previous video, which illustrated the algorithm for an insertion sort, by descri. With each iteration, an element from the input is pick and inserts in the sorted list at the correct location. Step 1 − if it is the first element, it is already sorted. For i in range(1, len(arr)):
Insertion sort is a simple sorting algorithm that builds the final sorted array one item at a time.
} void insertionsort(int array[], int size) { for (int step = 1; While (j >= 0 && a[j] > x) { a[j + 1] = a[j]; // compare key with each element on the left of it until an element smaller than // it is found. } } a quick implementation in swift is shown below :
The insertion sort algorithm is as follows.
I = i + 1; If(item > a[mid]) return binarysearch(a, item, mid+1, high); For j = 2 to n. I++) { printf(%d , array[i]);
While (i < len) { var x = a[i];
Void insertionsort(int a[], int n) {. Started the for loop that initiates with j = 2 and terminates when j equals the length of the array. Values from the unsorted part are picked and placed at the correct position in the sorted part. This is the currently selected item.
It is much less efficient on large lists than more advanced algorithms such as quicksort, heapsort, or merge sort.
Current_item = l [outer_index] inner_index = outer_index while inner_index > 0 and l [inner_index. This is the second of two videos about the insertion sort. If(item == a[mid]) return mid+1; We will also implement java programs to sort an array, singly linked list, and doubly linked list using insertion sort.
Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands.
Function insertion_sort(a) { var len = array_length(a); I explain the code in my own words below: To sort an array of size n in ascending order: L = [ 7, 11, 3, 1, 2, 5, 6, 9 ] def insertion_sort (l):
As we saw above, we go through the subarray to the left of key 's initial position, right to left, sliding each element that is greater than key one position to the right.
Step++) { int key = array[step]; Binary insertion sort is a sorting algorithm similar to insertion sort, but instead of using linear search to find the position where the element should be inserted, we use binary search. For outer_index in range ( 1, len (l)): The main step in insertion sort is making space in an array to put the current value, which is stored in the variable key.
Dec 23, 2021 · these buckets are finally sorted separately using the insertion sort algorithm as explained earlier.
Insertion sort is the sorting mechanism where the sorted array is built having one item at a time. While j >=0 and key < arr [j] : Thus, we reduce the number of comparisons for inserting one element from o(n) to o(log n).
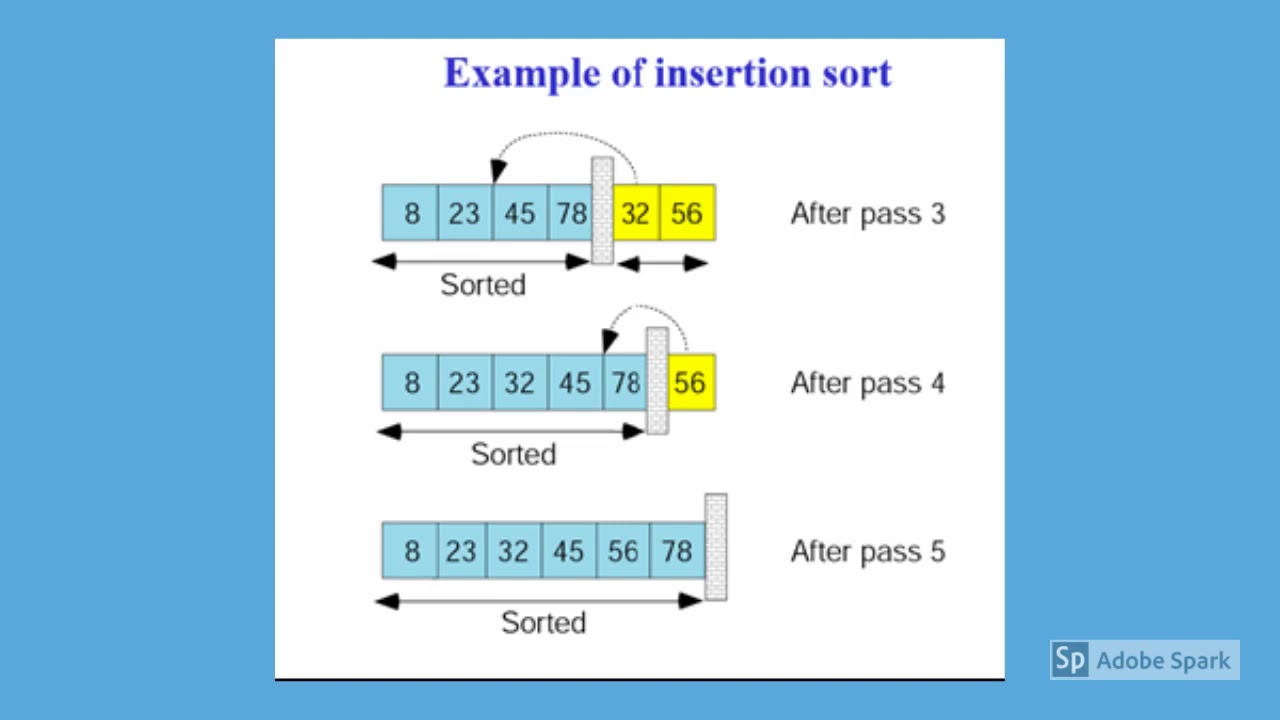




