In this tutorial, you will learn about insertion sort algorithm and its implementation in c, c++, java and python. I++) { int current = arr[i]; The insertion sort iterates through each element in the.
C Program for Insertion Sort
89 17 8 12 0
Insertion sort algorithm picks elements one by one and places it to the right position where it belongs in the sorted list of elements.
To sort an array of size n in ascending order: An insertion sort is a sorting technique used in c programming to sort elements of an array in ascending or descending order. In this sorting technique, we assume that the first number in the array is in the sorted section and the rest of all the other numbers in the array are in the unordered section. An insertion sort is a sorting technique used in c++ to sort elements of an array in ascending or descending order.
Insertion sort program in c++.
You can learn in depth about insertion sort algorithm from here; It is usually implemented when the user has a small data set. Insertion sort works by picking one element at a time and places it accordingly in the array. Insertion sort | logical programming in c | by mr.srinivas** for online training registration:
Before going through the program, lets see the steps of insertion sort with the help of an example.
Now we will pick each number from the unsorted. For (int i = 0; Insertion sort is a sorting algorithm that places an unsorted element at its suitable place in each iteration. Of elements in the list:\n);
Printf (\n please enter the array elements :
It is usually implemented when the user has a small data set. Insertion sort program in c.the basic idea of insertion sort method is to place an unsorted element into its correct position in a growing sorted list of. We assume that the first card is already sorted then, we select an unsorted card. Int n = sizeof(arr) / sizeof(arr[0]);
The idea behind the insertion sort is that first take one element, iterate it through the sorted array.
You pick one card and try to place that card in its correct position. Implement the insertion sort for the std::vector container in c++. The basic idea of this method is to place an unsorted element into its correct position in a growing sorted list of elements. Following is the c program to sort the elements by using the insertion sort technique −.
The array is kind of split into a sorted part and an unsorted part.
Insertion sort is a sorting algorithm that helps in sorting objects of an array one by one. Printf (\n please enter the total number of elements : } arr[j + 1] = current; The array is virtually split into a sorted and an unsorted part.
Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands.
The insertion sort works like a cards game, if the next value is greater than the previous element then the value goes to the right side of the element, else moves to the left side of the element, in the same way, every element will be sorted. Insertion sort in c is a simple and efficient sorting algorithm, that creates the final sorted array one element at a time. C program for insertion sort to sort numbers. A value from the unsorted part is picked and placed into its correct position in the sorted part.
#include <stdio.h> int main () { int a [100], number, i, j, temp;
This article will demonstrate how to implement an insertion sort algorithm in c++. } } int main() { int arr[] = {13,46,24,52,20,9}; Void insertionsort (int arr [], int n) {. The insertion sort is useful for sorting a small set of data.
To sort an array using insertion sort technique in c++ programming, you have to ask from user to enter the size for an array and then enter elements of that size in random order.
While (j >= 0 && arr[j] > current) { //swap arr[j + 1] = arr[j]; The basic idea of this method is to place an unsorted element into its correct position in a growing sorted list of elements. But, it is impractical to sort large arrays. Introduction to insertion sort in c.
Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time.
Insertion sort program in c++. In this guide, we will show you how to implement the insertion sort as a separate function that takes a reference to the std::vector object and modifies the contents in place. Jul 20, 2019 · insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands. Insertion sort is less efficient than the other sorting.
Insertion sort is just like how to sort playing cards in your hands.
Although it is simple to use, it is not appropriate for large data sets as the time complexity of insertion sort in the average case and worst case is o(n 2), where n is the number of items. In this sorting technique, we assume that the first number in the array is in the sorted section and the rest of all the other numbers in the array are in the unordered section. With a little modification, it will arrange numbers in descending order. I++) { scanf (%d, &a[i]);
This code implements insertion sort algorithm to arrange numbers of an array in ascending order.
After receiving the inputs, sort the given array in ascending order using insertion sort as shown in the program given below: It sorts smaller arrays faster than any other sorting algorithm. Now we will pick each number from the unsorted section and insert that number at a. Values from the unsorted part are picked and placed at the correct position in the sorted part.
The same approach is applied in insertion sort.
Insertion sort works similarly as we sort cards in our hand in a card game. This insertion sort in c program allows the user to enter the array size and the one dimensional array row elements. #include<stdio.h> void insertion_sort(int arr[], int n) { // insertion sort int i; An array of data, and the total number in the array.
#include<stdio.h> int main() { int a[50], i,j,n,t;
It will keep working on single elements and eventually put them in the right position, eventually ending with a sorted array. Best case complexity of insertion sort is o (n), average and the worst case complexity is o (n 2 ). In the following c program we have implemented the same logic.




![C++ Program to implement insertion sort [DEVCPP/GCC] TECHCPP](https://2.bp.blogspot.com/-oTwWUmftAss/V8bV-ZrmiZI/AAAAAAAAByg/aIBPQDfbRQQ1hw2i6W9IMHJ5yRSULz-cgCLcB/s1600/Slide4_Fotor.jpg)

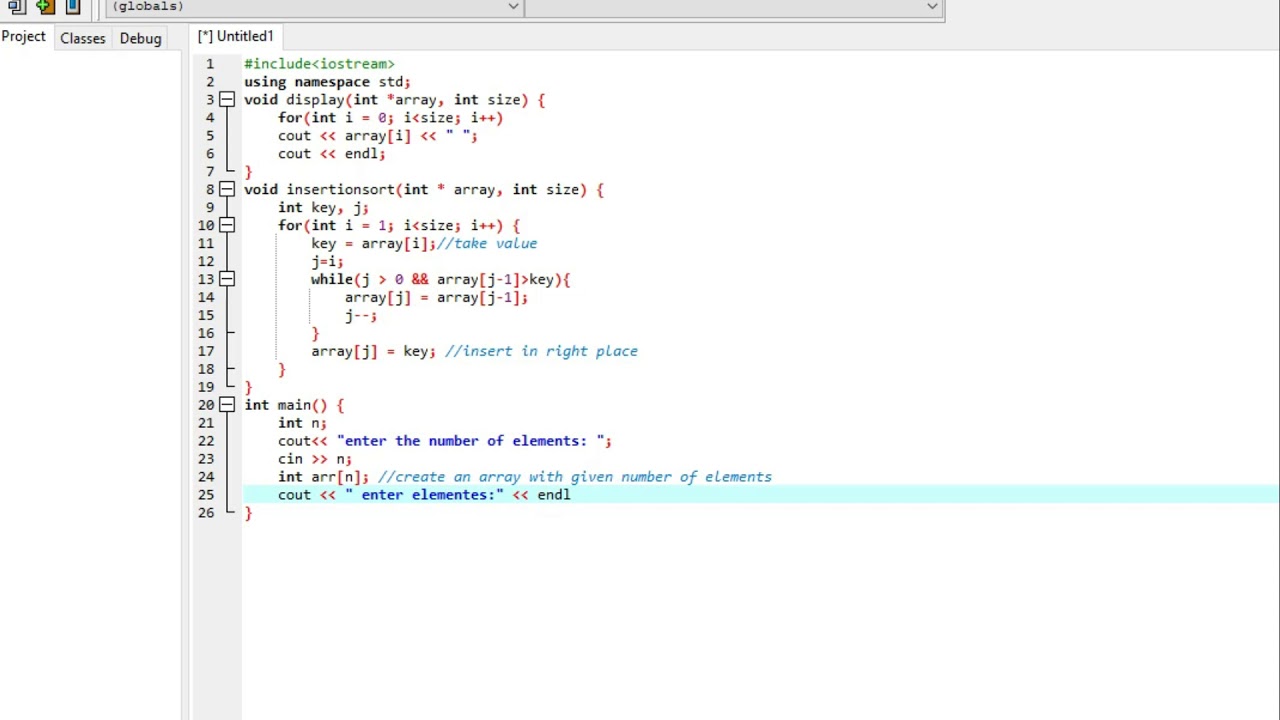