I++) { printf(%d , array[i]); Insertion sort works perfectly for small data sets, but it takes time when the data set is large. Void insertionsort (int arr [], int n)
Insertion sort implementation in C Sorting an integer
Let us take a closer look at below code.
Easiest to implement and to code.
O(1) input − the unsorted list: The time complexity of the insertion sort algorithm in the best case scenario is o(n), as no sorting would be required if the array is already sorted. // arrays to store time duration. Although it is simple to use, it is not appropriate for large data sets as the time complexity of insertion sort in the average case and worst case is o(n 2), where n is the number of items.
// well the sorting algorith time complexity is o (n^2) // we can see the nested for loop void insertionsort ( int arr [], int size) // or int *array { // size is.
At first look, it seems like insertion sort would take o (n 2) time, but it actually takes o (n) time. Ii) requires constant memory o(1). The time measured are only of insertion sort code and i/o operations are independent of it. Following is the c program to sort the elements by using the insertion sort technique −.
Therefore overall time complexity of the insertion sort is o (n + f (n)).
O(n 2) how insertion sort works? It is inefficient for large data set. O(n 2) worst case : The auxiliary space used by the iterative version is o(1) and o(n) by the recursive version for the call stack.
The average case scenario of this algorithm occurs when the array elements are in random order.
An array of data, and the total number in the array. I = 0 && arr [j] > key) { arr [j+1] = arr [j]; The time complexity is o(n^2). All those coders who are working on the c++ based application and are stuck on what is time complexity of insertion sort can get a collection of related answers to their query.
Big o of n in best case scenario.
15.384 seconds whereas in java 10000: Time & space complexity of insertion sort. /* function to sort an array using insertion sort*/ void insertionsort (int arr [], int n) { for (int i = 1; For most efficient algorithm as per time complexity, you can use heap or merge sort.
C program for insertion sort is very basic and easy to implement.
Insertion sort takes maximum time to sort if elements are sorted in reverse order. Insertion sort builds the sorted sequence one element at a time. 9 23 45 55 71 80 algorithm insertionsort(array, size) input: Not the fastest sorting algorithm.
The best case happens when the array is already sorted.
The while loop executes only if i > j and arr [i] < arr [j]. Printf (a_size, bubble, insertion, selection\n); Time complexity is the amount of time taken by a set of codes or algorithms to process or run as a function of the amount of input. (o(n^2) in all three cases.) note:
Insertion sort is less efficient than the other sorting.
While (it++ < 10) {. And it takes minimum time (order of n) when elements are already sorted. } } if we take a closer look at the insertion sort code, we can notice that every iteration of while loop reduces one inversion. It has very high time complexity.
O(n) for best case, o(n2) for average and worst case.
Assuming all possible inputs are equally likely, evaluate the average, or expected number c i of comparisons at each stage i = 1;:::;n 1. Advantages of this selection sort: I++) { scanf (%d, &a[i]); Less than 1 minute read.
} arr [j+1] = key;
Long int n = 10000; The complexity of insertion sort technique. Therefore, it is an example of an incremental algorithm. The time complexity of insertion sort is o(n^2).
The time complexity of insertion sort.
The best case happens when the array is already sorted. #include<stdio.h> int main() { int a[50], i,j,n,t; Double tim1 [10], tim2 [10], tim3 [10]; And in the average or worst case scenario the complexity is of the order o (n 2 ).
{6, 5, 3, 1, 8, 4}
Long int a [n], b [n], c [n]; The same approach is applied in insertion sort. C program for insertion sort. Step++) { int key = array[step];
1.924 seconds my code is normal insertion sort code.
For insertion sort, the time complexity is of the order o (n) i.e. 9 45 23 71 80 55 output − array after sorting: } void insertionsort(int array[], int size) { for (int step = 1; Therefore total number of while loop iterations (for all values of i) is same as number of inversions.
Assuming all possible inputs are equally likely, evaluate the average, or expected number c i of comparisons at each stage i = 1;:::;n 1.
Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands. #include using std :: The complexity of an algorithm is the measure of the number of comparisons made in the algorithm—an algorithm’s complexity measure for the worst case, best case, and the average case. Time complexity is o (n^2), we can see the nested for loop!
Insertion sort is used when number of elements is small.
// insertion sort in c #include <stdio.h> // function to print an array void printarray(int array[], int size) { for (int i = 0; Incremental approach sorting in place: Its time complexity is like best case is o(n) and average and worst case is o(n^2). Of elements in the list:\n);
Here is code for insertion sort in c++.
} arr [j+1] = key; But, it is not much efficient in terms of time complexity. Programmers need to enter their query on what is time complexity of insertion sort related to c++ code and they'll get their ambiguities clear immediately. // compare key with each element on the left of it until an element smaller than // it is found.
Calculate the average total number c= np1 i=1 i.
Insertion sort is a stable sorting technique because it does not change the relative order of elements. The idea behind the insertion sort is that first take one element, iterate it through the sorted array.
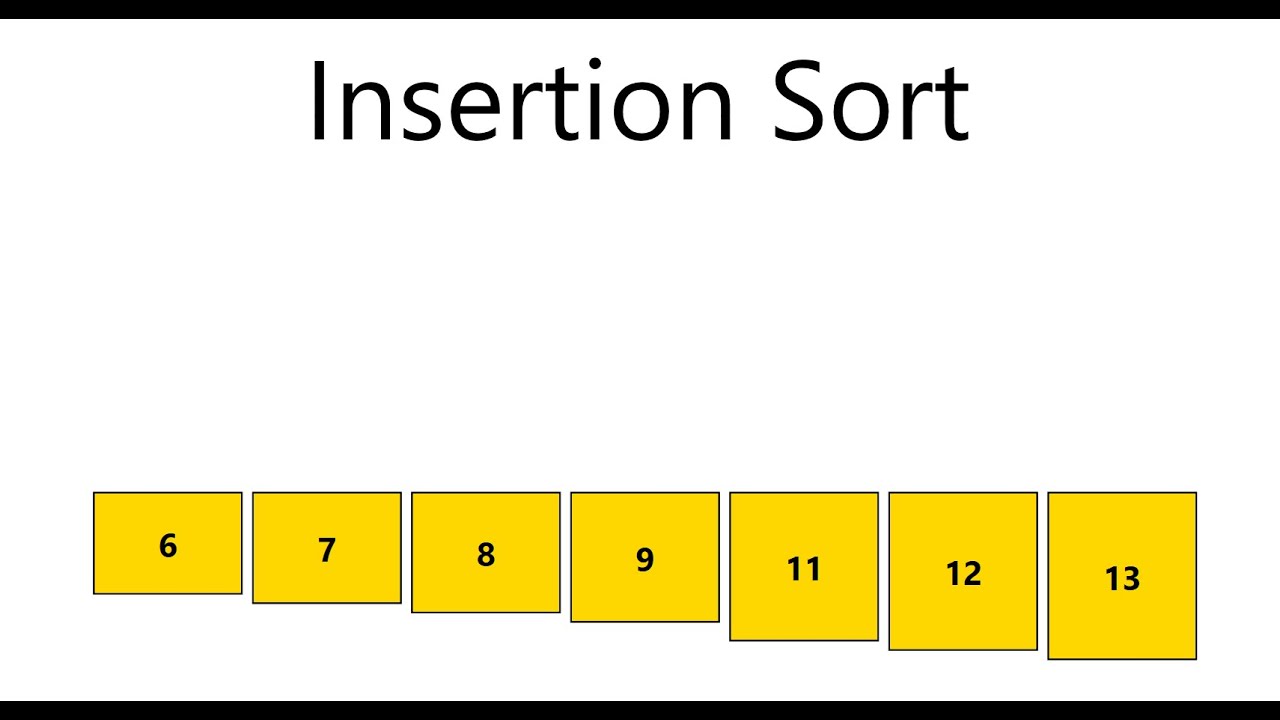





