I++) { int current = arr[i]; Insertion sort algorithm picks elements one by one and places it to the right position where it belongs in the sorted list of elements. Here we discuss how to perform insertion sort in c along with the example and output.
C++ Programs for Insertion Sort Studytonight
7 enter 5 element ::
1 enter 3 element ::
3) change head of given linked list to head of sorted (or result) list. 0 enter 6 element :: The same approach is applied in insertion sort. ‘array’ is a collection of variables of the same data type which are accessed by a single name.
The question is, write a program in c++.
Although it is simple to use, it is not appropriate for large data sets as the time complexity of insertion sort in the average case and worst case is o(n 2), where n is the number of items. Now we will pick each number from the unsorted section and insert that number at a. #include
89 17 8 12 0
Insertion sort in c++ ‘insertion sort’ uses the following algorithm to sort the elements of. By placing an unsorted element at its suitable location in each iteration. Step++) { int key = array[step]; Here we are going to learn how to implement insertion sort in c++.
It’s very useful with small data set or partially sorted data and not efficient if data is sorted in descending order and you want to sort data in.
Thus insertion sort is one of the simplest sorting methods which sorts all elements of an array. ‘sorting’ in programming refers to the proper arrangement of the elements of an array (in ascending or descending order). Of elements in the list:\n); However, insertion sort provides several advantages like simple implementation, efficient for (quite) small data sets, more efficient in practice than most other.
9 after insertion sort, sorted list is ::
An insertion sort is a sorting technique used in c programming to sort elements of an array in ascending or descending order. Please refer complete article on insertion sort for more details! Insertion sort is a sorting technique, which use to sort the data in ascending or descending order, like another sorting technique (selection, bubble, merge, heap, quicksort, radix, counting, bucket, shellsort, and comb sort). The idea behind the insertion sort is that first take one element, iterate it through the sorted array.
This insertion sort in c program allows the user to enter the array size and the one dimensional array row elements.
An array of data, and the total number in the array. //separate method to print the list provided by the calling method void printelems (vector < int > list) { int i; C program for insertion sort using for loop. Next, we are using nested for loop to sort the array elements using insertion sort.
0 1 2 4 6 7 9 process returned 0
Int arr [] = { 12, 11, 13, 5, 6 }; Following is the c program to sort the elements by using the insertion sort technique −. Int len = list.size (); It is much less efficient on large lists than more advanced algorithms such as quicksort, heapsort, or merge sort.
While (j >= 0 && arr[j] > current) { //swap arr[j + 1] = arr[j];
In this guide, we will show you how to implement the insertion sort as a separate function that takes a reference to the std::vector object and modifies the contents in place. } void insertionsort(int array[], int size) { for (int step = 1; Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time. In the above program, the compiler asks the user to enter the n elements and stored in the array arr.
The insertion sort iterates through each element in the.
} arr[j + 1] = current; // insertion sort in c #include <stdio.h> // function to print an array void printarray(int array[], int size) { for (int i = 0; 6 enter 7 element :: 1) create an empty sorted (or result) list 2) traverse the given list, do following for every node.
#include<stdio.h> void insertion_sort(int arr[], int n) { // insertion sort int i;
Printf(enter the number of elements :: .a) insert current node in sorted way in sorted or result list. Best case complexity of insertion sort is o (n), average and the worst case complexity is o (n 2 ). In this sorting technique, we assume that the first number in the array is in the sorted section and the rest of all the other numbers in the array are in the unordered section.
Insertion sort program in c++.
} } int main() { int arr[] = {13,46,24,52,20,9}; 7 enter elements to the array :: /* c++ program to implement insertion sort using array */ enter size of array :: Printf(the sorted elements are ::
After receiving the inputs, sort the given array in ascending order using insertion sort as shown in the program given below:
This article will demonstrate how to implement an insertion sort algorithm in c++. For (int i = 0; 5 6 11 12 13. Do the same for the remaining for loop iterations of the one.
4 enter 2 element ::
/** insertion sort **/ #include <stdio.h> int main (void) { int size, array[80], i, j, element; 2 enter 4 element :: C program for insertion sort to sort numbers. The basic idea of this method is to place an unsorted element into its correct position in a growing sorted list of elements.
I++) { temp = a [i];
Int len = a.size (); Insertion sort program in c.the basic idea of insertion sort method is to place an unsorted element into its correct position in a growing sorted list of. } //the insertion sort logic void insertionsort (vector < int > & a) { int i, loc, temp; #include #include using namespace std;
To sort an array using insertion sort technique in c++ programming, you have to ask from user to enter the size for an array and then enter elements of that size in random order.
// compare key with each element on the left of it until an element smaller than // it is found. I++) { element = array[i]; Then we are comparing those elements so they can be arranged properly using. Below is simple insertion sort algorithm for linked list.
Implement the insertion sort for the std::vector container in c++.
I++) { printf(%d , array[i]); This is a guide to insertion sort in c. Insertion sort is less efficient than the other sorting. Insertion sort algorithm is one of the simplest algorithms, effective for simple data sets.
Before going through the program, lets see the steps of insertion sort with the help of an example.
With a little modification, it will arrange numbers in descending order. This algorithm builds the sorted array by one element at a time, i.e. I++) cout << list [i] << ; } for (i = 0;
This code implements insertion sort algorithm to arrange numbers of an array in ascending order.
Int n = sizeof(arr) / sizeof(arr [0]); Int n = sizeof(arr) / sizeof(arr[0]); How to implement insertion sort for linked list?

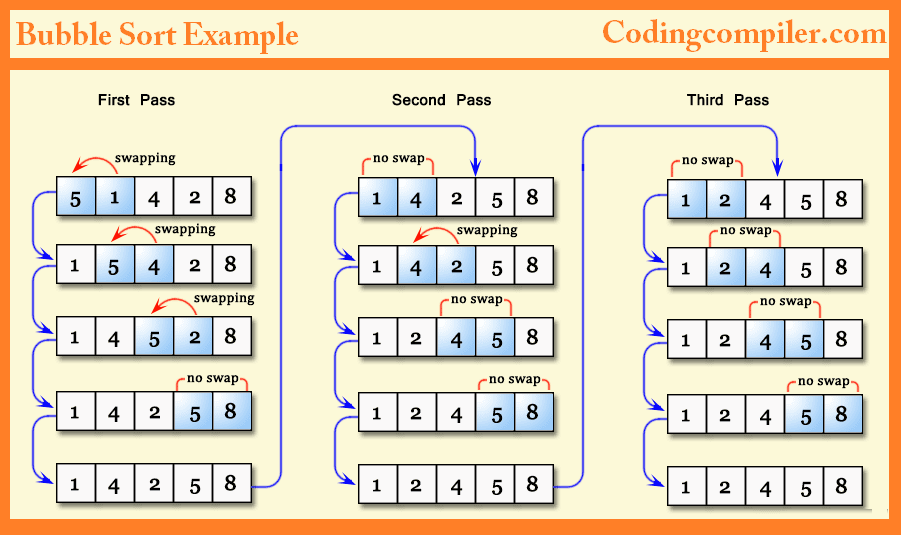




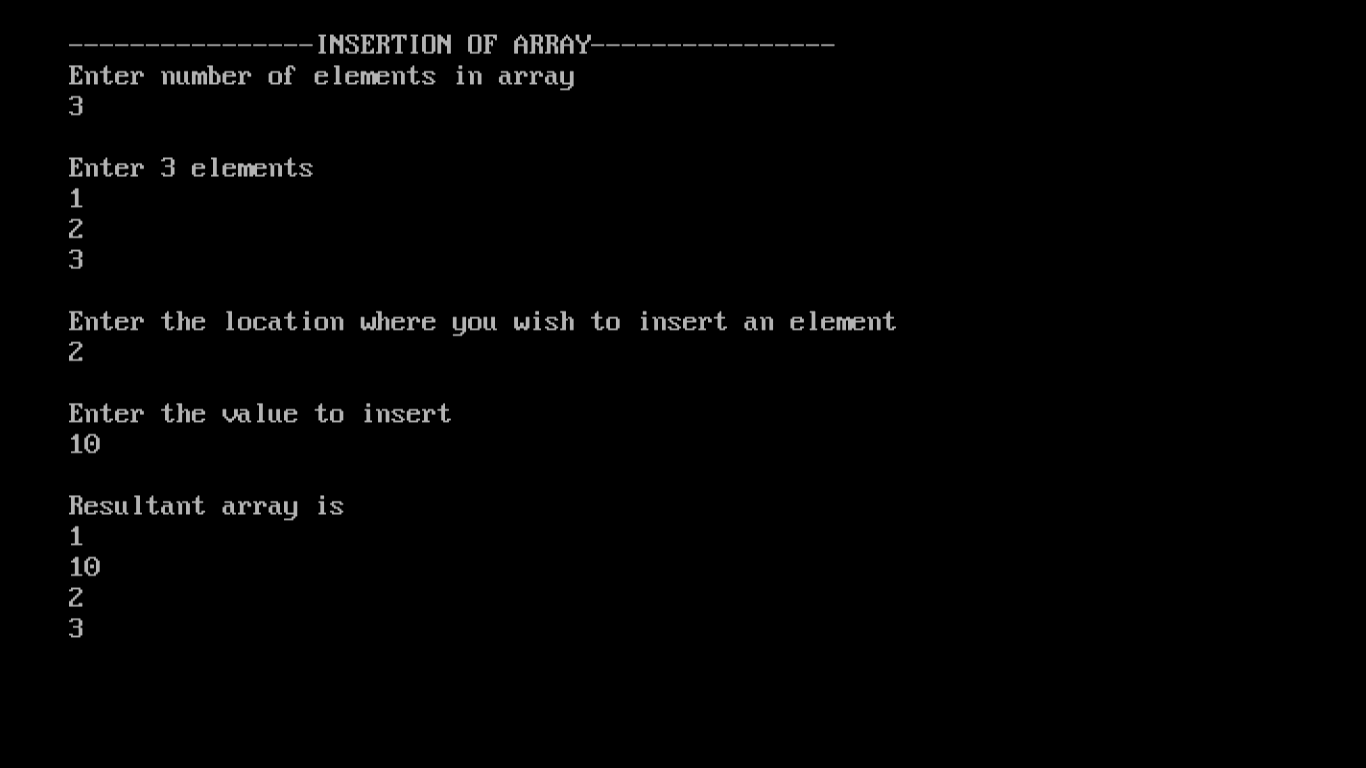