With a little modification it will arrange numbers in descending order. The answer to this question is: #include using namespace std;
C Program for Insertion Sort
The basic idea of this method is to place an unsorted element into its correct position in a growing sorted list of elements.
/* c++ program to implement insertion sort using array */ enter size of array ::
This c program will show you how to short numbers at the time of insertion. C program for insertion sort to sort numbers. Next, we are using nested for loop to sort the array elements using insertion sort. Hence in order to sort the array using pointers, we need to access the elements of the array using (pointer + index) format.
9 after insertion sort, sorted list is ::
An insertion sort is a sorting technique used in c++ to sort elements of an array in ascending or descending order. } void insertionsort(int array[], int size) { for (int step = 1; } void insertion (int* l, int. /* c program to implement insertion sort using arrays */ #include
#include <stdio.h> int main () { int a [100], number, i, j, temp;
Starting from the second element, we compare it with the first element and swap it if it is not in order. Although it is simple to use, it is not appropriate for large data sets as the time complexity of insertion sort in the average case and worst case is o(n 2 ) , where n is the number of items. For (int x = 0; Scanf ( %d , &size);
1 enter 3 element ::
Insertion sort is a very efficient algorithm for sorting smaller arrays. After receiving the inputs, sort the given array in ascending order using insertion sort as. Below is the implementation of the above approach: 4 enter 2 element ::
The logic we use to sort the array elements in ascending order is as follows −.
Show activity on this post. This allows the user of the program to enter arbitrarily long text which can overwrite all of the programs memory. Insertion sort is similar to arranging the documents of a bunch of students in order of their ascending roll number. Ask question asked 4 years, 7 months ago.
Here is a c program that takes the elements of an array as input, sort it using insertion sort algorithm and prints.
Following is the c program to sort an array in an ascending order −. Later on, i'll explain each and every steps involved in this program. Int n = sizeof(arr) / sizeof(arr [0]); I++) { temp = a[i];
Insertion sort using pointer to an array.
This insertion sort in c program allows the user to enter the array size and the one dimensional array row elements. 7 enter 5 element :: 6 enter 7 element :: C program to insertion sort using array.
0 enter 6 element ::
Please refer complete article on insertion sort for more details! // insertion sort in c #include <stdio.h> // function to print an array void printarray(int array[], int size) { for (int i = 0; ++i){ for (j = i + 1; Best case complexity of insertion sort is o(n), average and the worst case complexity is o(n 2).
Printf ( enter array size:
Insertion sort program in c++. Implement the insertion sort for the std::vector container in c++. For (int i = 0; Sorting an array using insertion sort in c.
Insertion sort is a sorting algorithm that helps in sorting objects of an array one by one.
This article will demonstrate how to implement an insertion sort algorithm in c++. // compare key with each element on the left of it until an element smaller than // it is found. Sorting an array of strings in c using insertion sort. C program for insertion sort using for loop.
Step++) { int key = array[step];
The insertion sort iterates through each element in the. An array of data, and the total number in the array. #include<stdio.h> #include<conio.h> int main () { int arr [50], size, i, j, k, element, index; In this guide, we will show you how to implement the insertion sort as a separate function that takes a reference to the std::vector object and modifies the contents in place.
0 1 2 4 6 7 9 process returned 0
With a little modification, it will arrange numbers in descending order. This code implements insertion sort algorithm to arrange numbers of an array in ascending order. The array can be fetched with the help of pointers with the pointer variable pointing to the base address of the array. 5 6 11 12 13.
Int main () { int a [6] = {9, 5, 3, 7, 5, 6};
This code implements insertion sort algorithm to arrange numbers of an array in ascending order. I++) { printf(%d , array[i]); The idea behind the insertion sort is that first take one element, iterate it through the sorted array. X[0] may be considered as a sorted array of one element.
Modified 3 years, 11 months ago.
Printf ( enter %d array. Insertion sort works by picking one element at a time and places it accordingly in the array. I++) { printf(\nenter the element [ %d ].: 2 enter 4 element ::
It will keep working on single elements and eventually put them in the right position, eventually ending with a.
In this sorting technique, we assume that the first number in the array is in the sorted section and the rest of all the other numbers in the array are in the unordered section. Printf(\nenter the number of terms.: The question is, write a program in c that sorts any given array in ascending order using insertion sort technique. Cout << now insertion sort \n;
Let x is an array of n elements.
Int arr [] = { 12, 11, 13, 5, 6 }; Printf(\nenter the elements of the array.: While(temp<a[j] && j>0) { a[j+1] = a[j]; To sort an array using insertion sort technique in c++ programming, you have to ask from user to enter the size for an array and then enter elements of that size in random order.
Printf (\n please enter the total number of elements :
I++) cout << a [i] << ; X++) cout << a [x] << \n; Viewed 8k times 3 \$\begingroup\$ i am a java programmer who has c this semester. 7 enter elements to the array ::
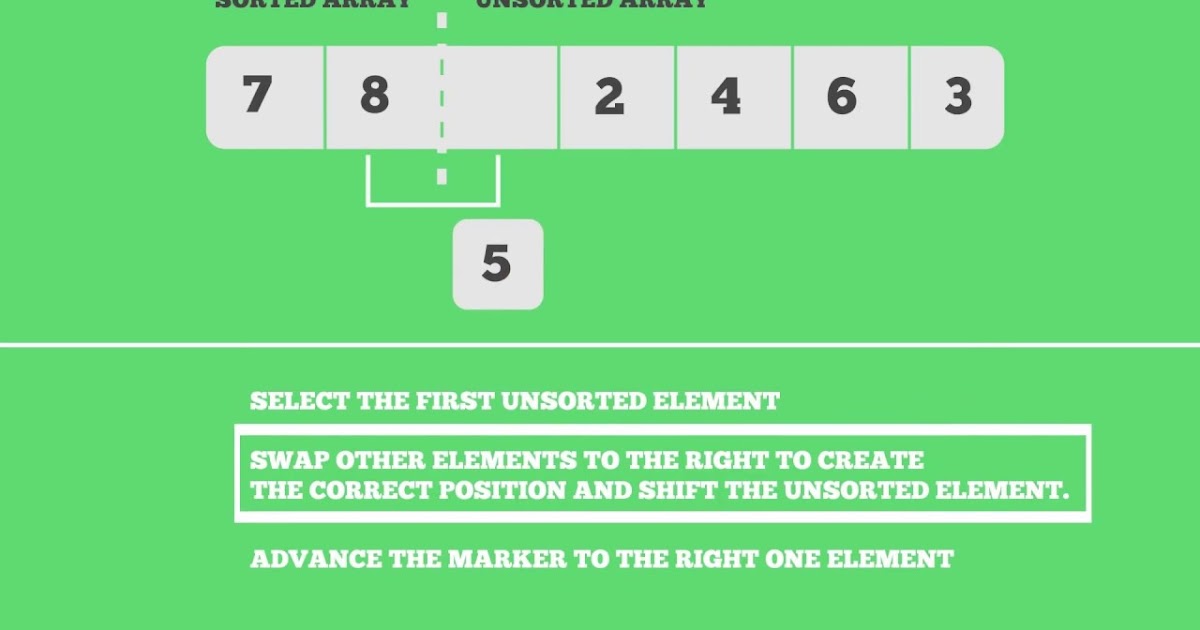

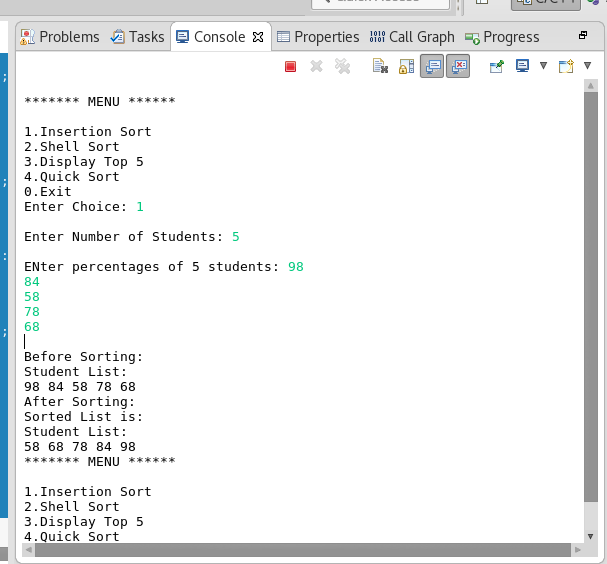


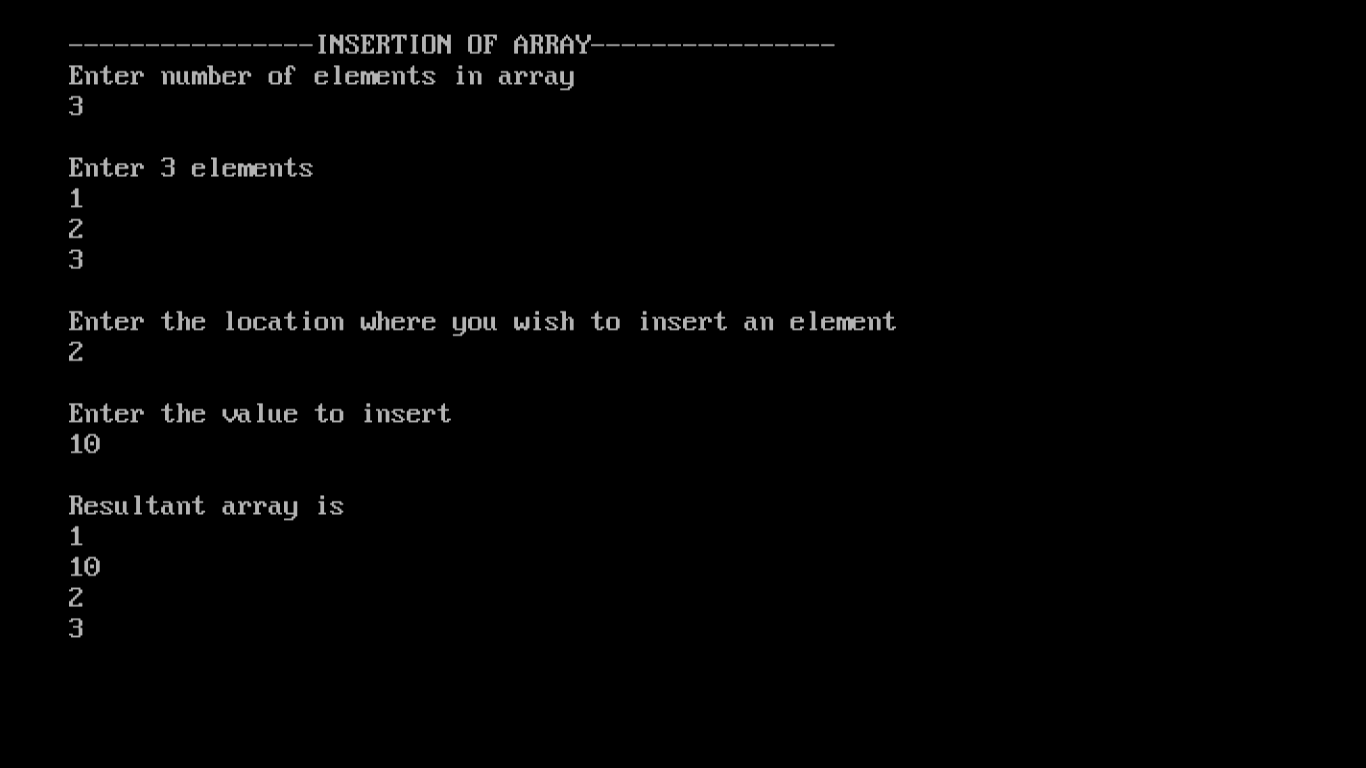
