Here n is the number of elements in the list. For (int j = 1; To sort an array of size n in ascending order:
Insertion Sort in Java Example code algorithm YouTube
Insertion sorting time complexity is o(n^2).
Random random = new random ();
For ( int i=0;i<size;i++) {. Int key = arr [i]; For ( int j = 1; ( array [i] > key ) ) { array [i+1] = array [i];
Algorithm is prepared based array and array index starts from 0.
It will keep working on single elements and eventually put them in the right position, eventually ending with a. Insertion sort works by picking one element at a time and places it accordingly in the array. Public class main { public static void insertionsort(int array []) { int n = array.length; J++) { int key = array [j];
} array [i+ 1] = key;
Step++) { int key = array[step]; Insertion sort is a simple sorting algorithm that builds the final sorted array or list one item at a time. } } public static void main (string a []) { int [] arr1 = {9,14,3,2,43,11,58,22}; Otherwise, it will be placed at the left side.
Here is my arraylist it is filled with random integers:
// insertion sort in java import java.util.arrays; Void sort (int arr []) {. Insertionsort (myarray);//sorting array using insertion sort system.out.println (after insertion. It is assumed that the first card is already sorted in the card game, and then we select an unsorted card.
The insertion sort algorithm is as follows.
How insertion sort works in insertion sort you take one element at a time and the elements on the left side of the current element are considered temporarily sorted for example if you are at 4th index then. For (int j = 1; It is much less efficient on large lists than more advanced algorithms such as quicksort, heapsort, or merge sort. Insertion sort is a sorting algorithm that helps in sorting objects of an array one by one.
Compare the current element (key) to its predecessor.
For (int step = 1; Try { bufferedreader obj= new bufferedreader ( new inputstreamreader (system. /* java program to implement insertion sort */ public class insertionsort { public static void insertionsort (int arr []) { int temp; This article covers a program in java that sorts an array using insertion sort technique.
Now we will pick each number from the unsorted section and insert that number at a proper position.
Int key = array [j]; Code for program of insertion sort in java. Here is an example with an array of length 4 to understand insertion sort algorithm. Introduction insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a.
Following is the java program for recursive insertion sort −.
Publicvoid getdata () { system. While (j >= 0 && my_arr[j] > last){ my_arr[j+1] = my_arr[j]; I++) { temp = arr [i]; J++) { int key = array[j];
Class insertionsort { void insertionsort(int array[]) { int size = array.length;
Public class insertionsort { public static void insertionsort (int array []) { int n = array.length; 4 is compared with element on its left which is 6. Array [i+1] = array [i]; } } public static void main(string.
Public class insertionsortexample { public static void main (string a []) { int [] myarray = {860,8,200,9};
Insertion sorting java program this java program is used to sort the array of integers using insertion sorting technique. For (int j = 1; In this sorting technique, we assume that the first number in the array is in the sorted section and the rest of all the other numbers in the array are in the unordered section. We will also implement java programs to sort an array, singly linked list, and doubly linked list using insertion sort.
Insertion sort algorithm is good for small elements only because it requires more time for sorting the large number of elements.
Java program for insertion sort. // compare key with each element on the left of it until an element smaller than // it is found. At each iteration, insertion sort removes one element from the input data,. Arraylist oh = new arraylist ();
Out.print ( enter how many data you want to enter :
Suppose the passed array is [6, 4, 2, 9]. Public class demo{ static void recursive_ins_sort(int my_arr[], int arr_len){ if (arr_len <= 1) return; It iterates, take one input element each repetition, and growing a sorted output list. } array [i+1] = key;
If the key element is smaller than its predecessor, compare it to the elements before.
An insertion sort is a sorting technique used in java to sort elements of an array in ascending or descending order. } } public static void main(string a[]) { int arr[] = {21,60,32,01,41,34,5}; Public class tester { public static void insertionsort(int array[]) { int n = array.length; Java program example to sort an array using insertion sort algorithm:
J++) { int key = array [j];
Insertion sort works similar to the sorting of playing cards in hands. If the selected unsorted card is greater than the first card, it will be placed at the right side; While (j >= 0 &&. Let’s write a java code which implements insertion sort algorithm.
Public static void insertionsort (int array []) {.
Insertion sort program in java. //traverse an array from left to right for (int i = 1; System.out.println (array before insertion sort); Java program to insertion sort with example 1.
Class sort { string str;
How do you sort an arraylist using insertion? Since 4 is smaller so it has to be inserted at the index 0. Out of the three simpler sorting algorithms insertion sort, selection sort and bubble sort, insertion sort is considered a better option in most of the scenarios.




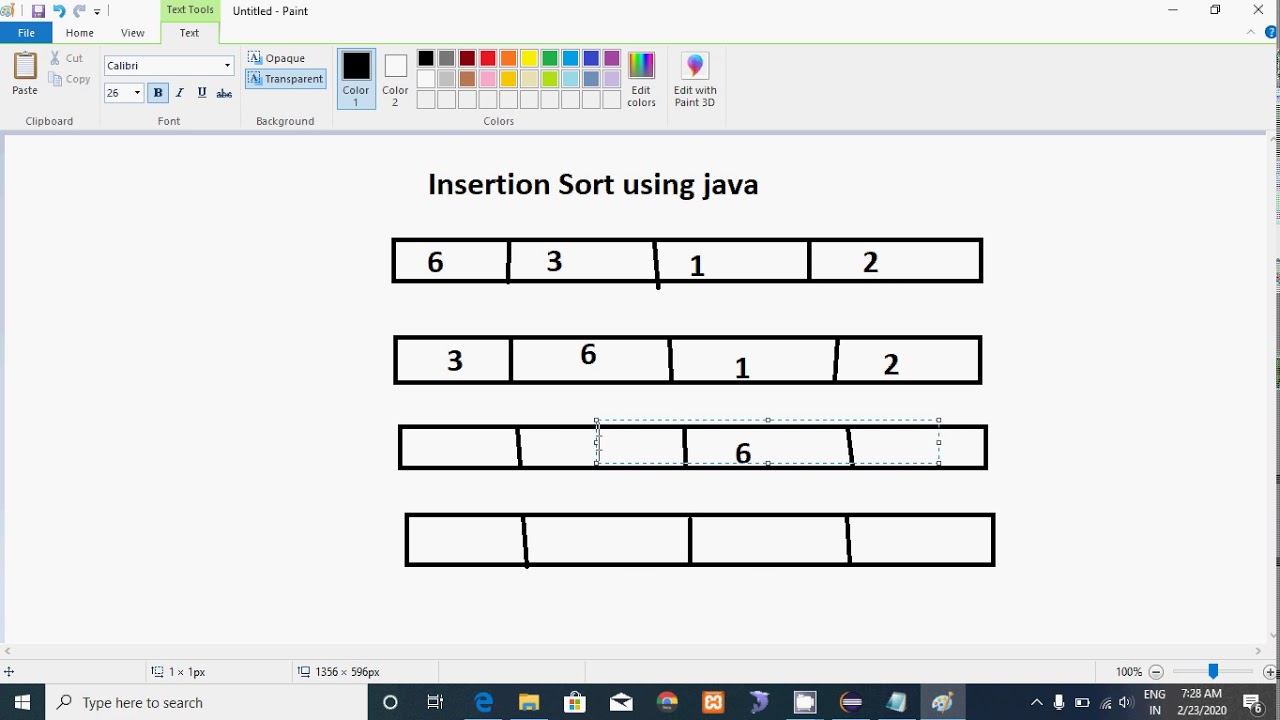
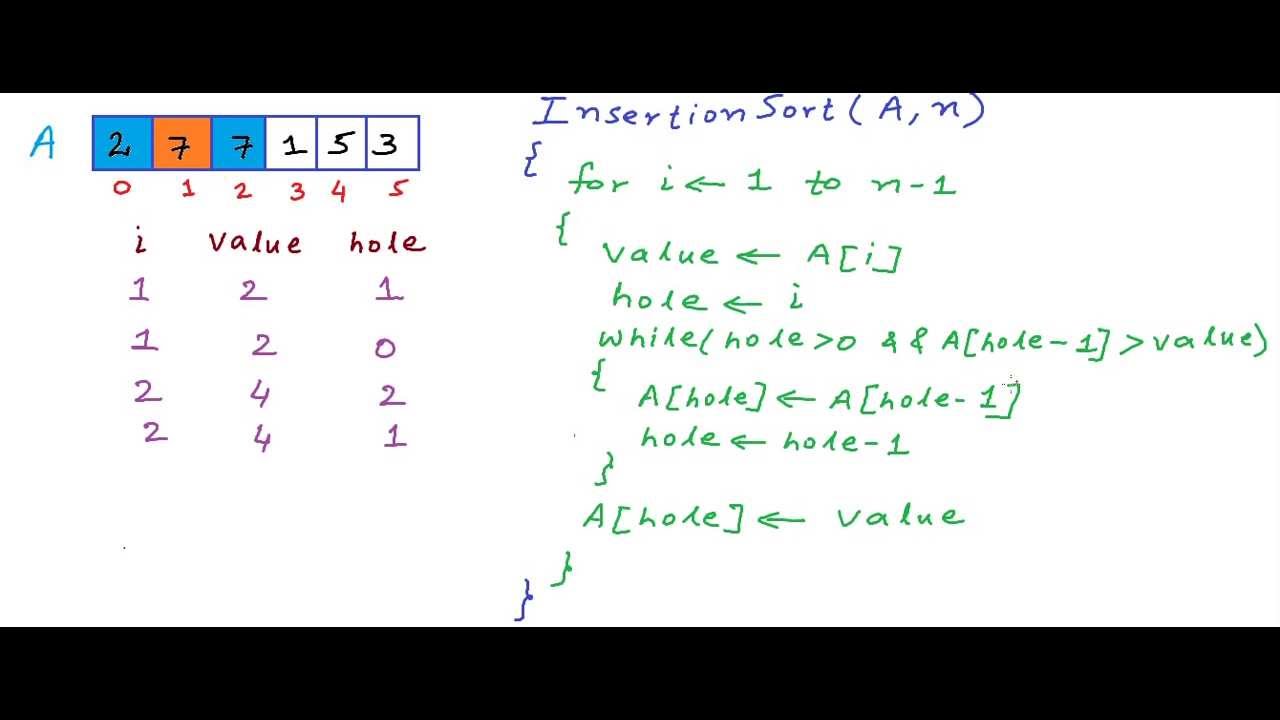
