This article will demonstrate how to implement an insertion sort algorithm in c++. This code implements insertion sort algorithm to arrange numbers of an array in ascending order. I++) { for(j = i;
sorting programs in c insertion sort, bubble sort
An insertion sort is a sorting technique used in c programming to sort elements of an array in ascending or descending order.
Now we will pick each number from the unsorted section and insert that number at a.
Introduction to insertion sort in c++. With a little modification, it will arrange numbers in descending order. #include<stdio.h> int main() { int a[50], i,j,n,t; } void insertionsort(int array[], int size) { for (int step = 1;
Of elements in the list:\n);
1) create an empty sorted (or result) list 2) traverse the given list, do following for every node. Next, we are using nested for loop to sort the array elements using insertion sort. Following is the c program to sort the elements by using the insertion sort technique −. I++) { printf(%d , array[i]);
Compare the new picked element with the sorted element of an array.
Printf(\nenter the total number of elements : .a) insert current node in sorted way in sorted or result list. Here we discuss how to perform insertion sort in c along with the example and output. Santosh shaw from india pointed out that an earlier version of this page did not use a correct insertion sort algorithm since it did not run in o(n).
The insertion sorting c++ is implemented by the use of nested loops, it works in a way.
// compare key with each element on the left of it until an element smaller than // it is found. Insertion sort program using functions in c /* c program for insertion sort using functions*/ #include <stdio.h> void insertion(int a[], int number) { int i, j, temp; It is more complex than insertion sort, but for long lists it is quicker. Below is simple insertion sort algorithm for linked list.
Void insertionsort (int arr [], int n)
// function to print an array void printarray(int array[], int size) { for (int i = 0; Now pick the new element which you want to insert. To sort an array using insertion sort technique in c++ programming, you have to ask from user to enter the size for an array and then enter elements of that size in random order. // insertion sort in c #include <stdio.h> // function to print an array void printarray(int array[], int size) { for (int i = 0;
This is an example c program demonstrating insertion sort.
} void insertionsort(int array[], int size) { for (int step = 1; Best case complexity of insertion sort is o (n), average and the worst case complexity is o (n 2 ). It is preferred our selection sort but other faster algorithms like bubble sort, quicksort, and merge sort are preferred our insertion sort. How to implement insertion sort for linked list?
C++ insertion sort example | insertion sort program in c++ is today’s topic.
After receiving the inputs, sort the given array in ascending order using insertion sort as shown in the program given below: #include <stdio.h> int main () { int a [100], number, i, j, temp; 3) change head of given linked list to head of sorted (or result) list. Insertion sort c++ is one of the most commonly used algorithm in c++ language for the sorting of fewer values or smaller arrays.
Printf (\n please enter the total number of elements :
It is much less efficient on large lists than more advanced algorithms such as quicksort, heapsort, or merge sort. // insertion sort in c++ #include using namespace std; The insertion sort iterates through each element in the. I++) { scanf (%d, &a[i]);
Insertion sort program in c /* c program for insertion sort */ #include<stdio.h> int main() { int a[100], n, i, j, element;
Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands. C program for insertion sort to sort numbers. C program for insertion sort using for loop. This is a guide to insertion sort in c.
In this guide, we will show you how to implement the insertion sort as a separate function that takes a reference to the std::vector object and modifies the contents in place.
Printf(\nenter the array elements : Thus insertion sort is one of the simplest sorting methods which sorts all elements of an array. Implement the insertion sort for the std::vector container in c++. The question is, write a program in c++.
If there is only one element or the first element in an array, it behaves as a sorted array.
I++) { for(j = i; However, insertion sort provides several advantages like simple implementation, efficient for (quite). /* for counting total number of iterations */ count++; This insertion sort in c program allows the user to enter the array size and the one dimensional array row elements.
I++) { cout << array[i] << ;
Step++) { int key = array[step]; In this sorting technique, we assume that the first number in the array is in the sorted section and the rest of all the other numbers in the array are in the unordered section. Insert the value if the correct position is found. C program for insertion sort.
Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time.
Simple insertion sort program /* simple insertion sort program in c*/ /* data structure programs,c array examples */ #include<stdio.h> #include<conio.h> #define max_size 5 void insertion(int[]); Int main() { int arr_sort[max_size], i, j, a, t; An array of data, and the total number in the array. Printf(\nenter %d elements for sorting\n, max_size);
// compare key with each element on the left of it until an element.
We are going to discuss insertion sort in c++, though this sorting technique is not that much efficient in comparison to other sorting algorithms like quicksort, mergesort, selectionsort, heapsort, it is suitable for a simple set of data like quadratic sorting algorithms, etc. Royal52 aug 22, 2015 4849 0.
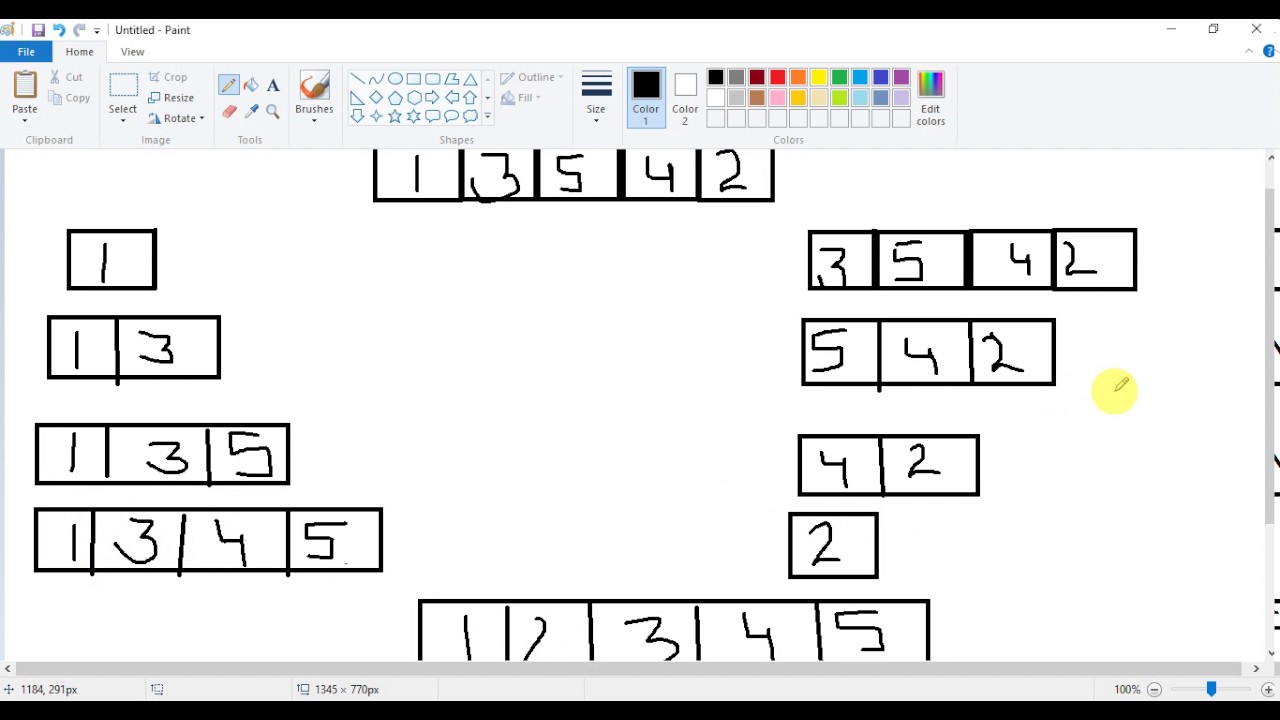
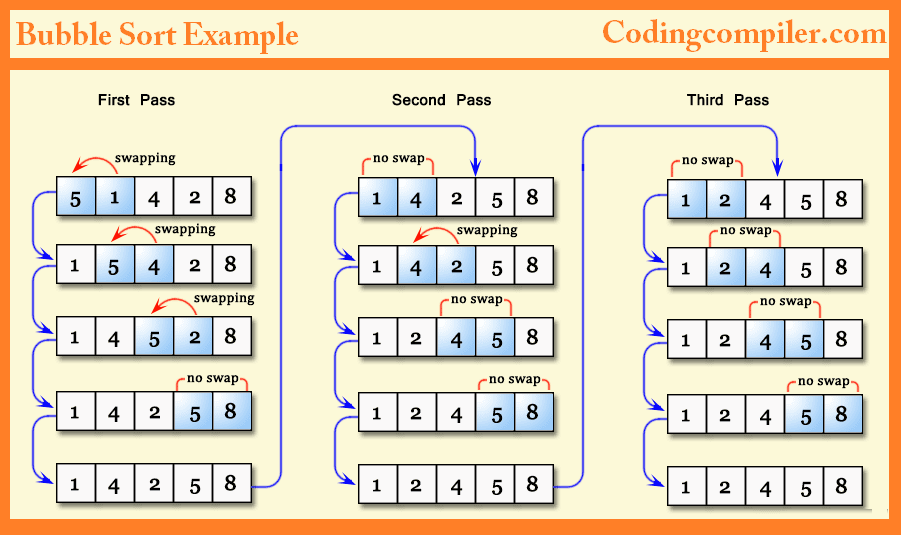




