Therefore an array of strings is an array of arrays of chars (so your insertionsort signature is wrong). Show activity on this post. This article will demonstrate how to implement an insertion sort algorithm in c++.
C Program for insertion sorting in C (With explanation
C program for insertion sort to sort numbers.
Void insertionsort (int v [], int s) { int i;
Before going through the program, lets see the steps of insertion sort with the help of an example. With a little modification, it will arrange numbers in descending order. This code implements insertion sort algorithm to arrange numbers of an array in ascending order. Printf (\n please enter the array elements :
A few problems with the original code:
Of elements in the list:\n); It can also be useful when the input element is almost sorted, only a few elements are misplaced in a big array. I++) { printf(%d , array[i]); Following is the c program to sort the elements by using the insertion sort technique −.
I++) { value = v [i];
1) you cannot copy strings using =; Compare the current element (key) to its predecessor. It’s more efficient with the partially sorted array or list, and worst with the descending order array and list. // compare key with each element on the left of it until an element smaller than // it is found.
Although it is simple to use, it is not appropriate for large data sets as the time complexity of insertion sort in the average case and worst case is o(n 2), where n is the number of items.
Another advantage of insertion sort is that it is a stable sort which means it maintains the order of equal elements in the list. Complexity analysis of the insertion sort algorithm. To sort an array of size n in ascending order: In particular today i was trying to understand the insertion sort.
// insertion sort in c #include <stdio.h> // function to print an array void printarray(int array[], int size) { for (int i = 0;
2) a string is an array of chars; An array of data, and the total number in the array. Introduction to insertion sort in c++. It is preferred our selection sort but other faster algorithms like bubble sort, quicksort, and merge sort are preferred our insertion sort.
Use strncpy for that (using = only assigns pointers).
Printf (\n please enter the total number of elements : Since this year i'm starting studying c programming at university. I++) { scanf (%d, &a[i]); The same approach is applied in insertion sort.
This insertion sort in c program allows the user to enter the array size and the one dimensional array row elements.
Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands. Next, we are using nested for loop to sort the array elements using insertion sort. In the following c program we have implemented the same logic. 89 17 8 12 0
Insertion sort c++ is one of the most commonly used algorithm in c++ language for the sorting of fewer values or smaller arrays.
The insertion sorting c++ is implemented by the use of nested loops, it works in a way. From the pseudo code and the illustration above, insertion sort is the efficient algorithm when compared to bubble sort or selection sort. In this guide, we will show you how to implement the insertion sort as a separate function that takes a reference to the std::vector object and modifies the contents in place. Best case complexity of insertion sort is o (n), average and the worst case complexity is o (n 2 ).
Insertion sort | logical programming in c | by mr.srinivas** for online training registration:
#include <stdio.h> int main () { int a [100], number, i, j, temp; Insertion sort is less efficient than the other sorting. I wrote this code that is perfectly working: The question is, write a program in c++ to implement.
After receiving the inputs, sort the given array in ascending order using insertion sort as shown in the program given below:
The insertion sort is useful for sorting a small set of data. The insertion sort iterates through each element in the. Iterate from arr [1] to arr [n] over the array. However, insertion sort works differently, instead of iterating through all of the data after every pass the algorithm only traverses the data it needs to until.
Insertion sort is the very simple and adaptive sorting techniques, widely used with small data items or data sets.
But, it is impractical to sort large arrays. Implement the insertion sort for the std::vector container in c++. To sort an array using insertion sort technique in c++ programming, you have to ask from user to enter the size for an array and then enter elements of that size in random order. Insertion sort is a faster and more improved sorting algorithm than selection sort.
Step++) { int key = array[step];
Royal52 aug 22, 2015 4849 0. #include<stdio.h> int main() { int a[50], i,j,n,t; } void insertionsort(int array[], int size) { for (int step = 1; If the key element is smaller than its predecessor, compare it to the elements before.
It sorts smaller arrays faster than any other sorting algorithm.
In selection sort the algorithm iterates through all of the data through every pass whether it is already sorted or not. Void insertionsort (int arr [], int n) {. Insertion sort algorithm picks elements one by one and places it to the right position where it belongs in the sorted list of elements. Note that c strings are null terminated, which simply means that a byte with the value of 0 signifies the end.
(j >= 0) && (value < v.
Some characteristics of insertion sort in c++. It is used mainly when the number of elements is small.
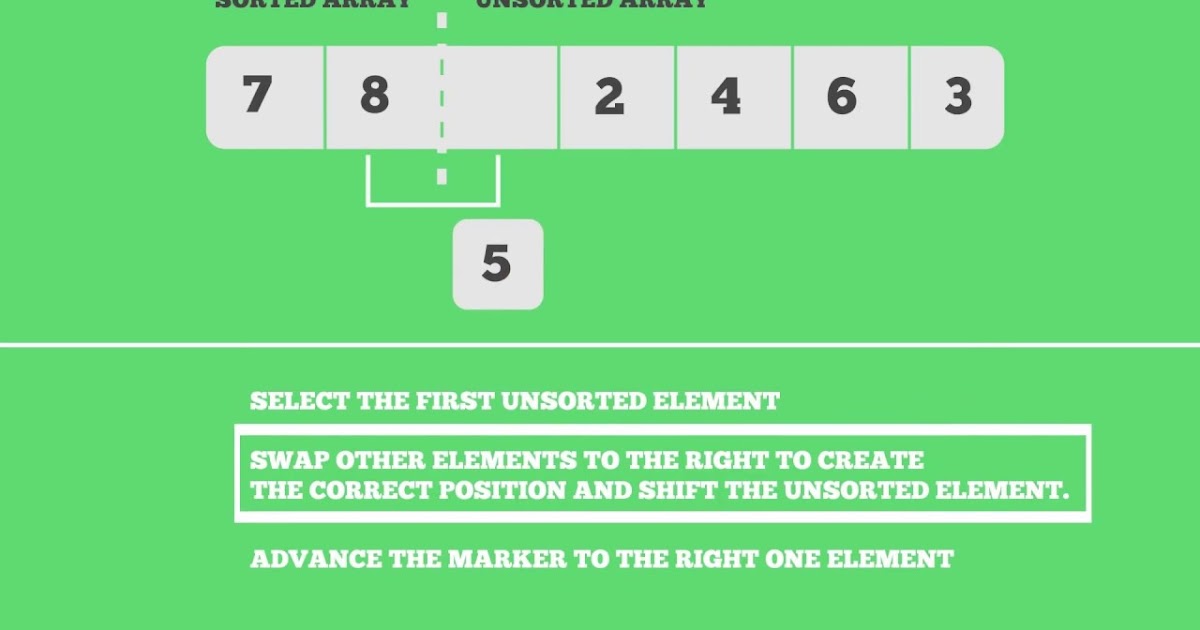
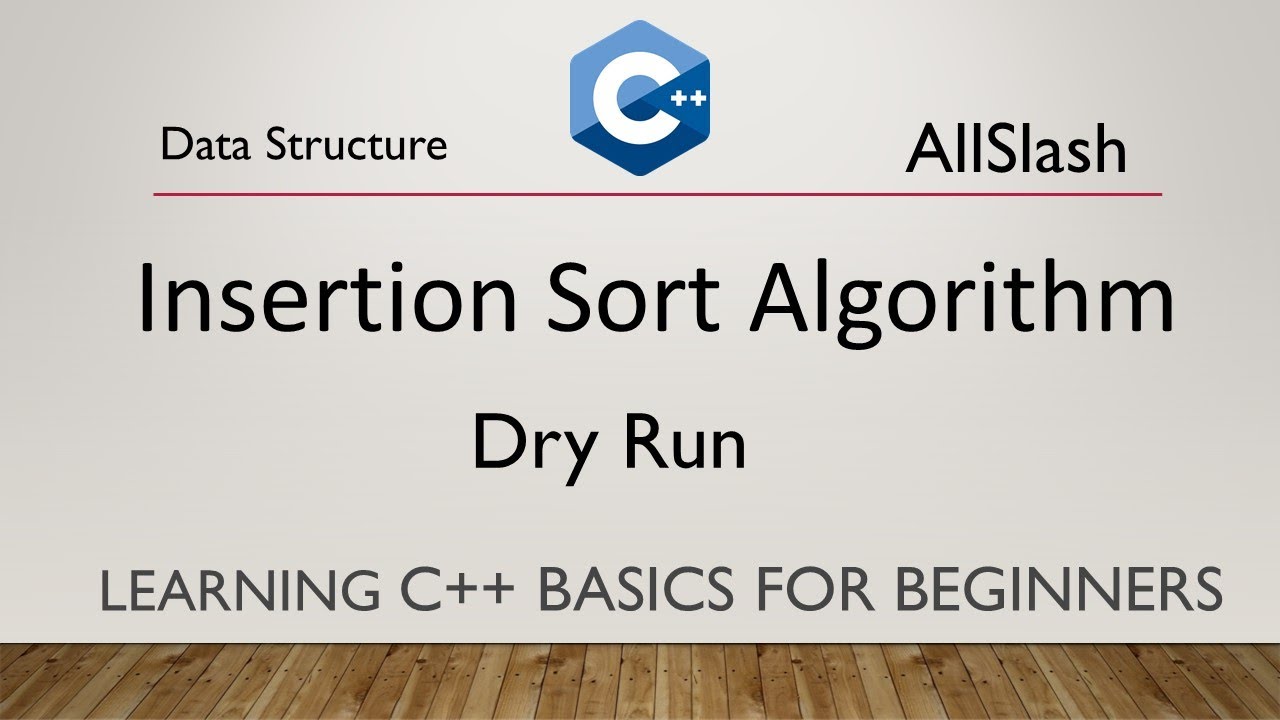

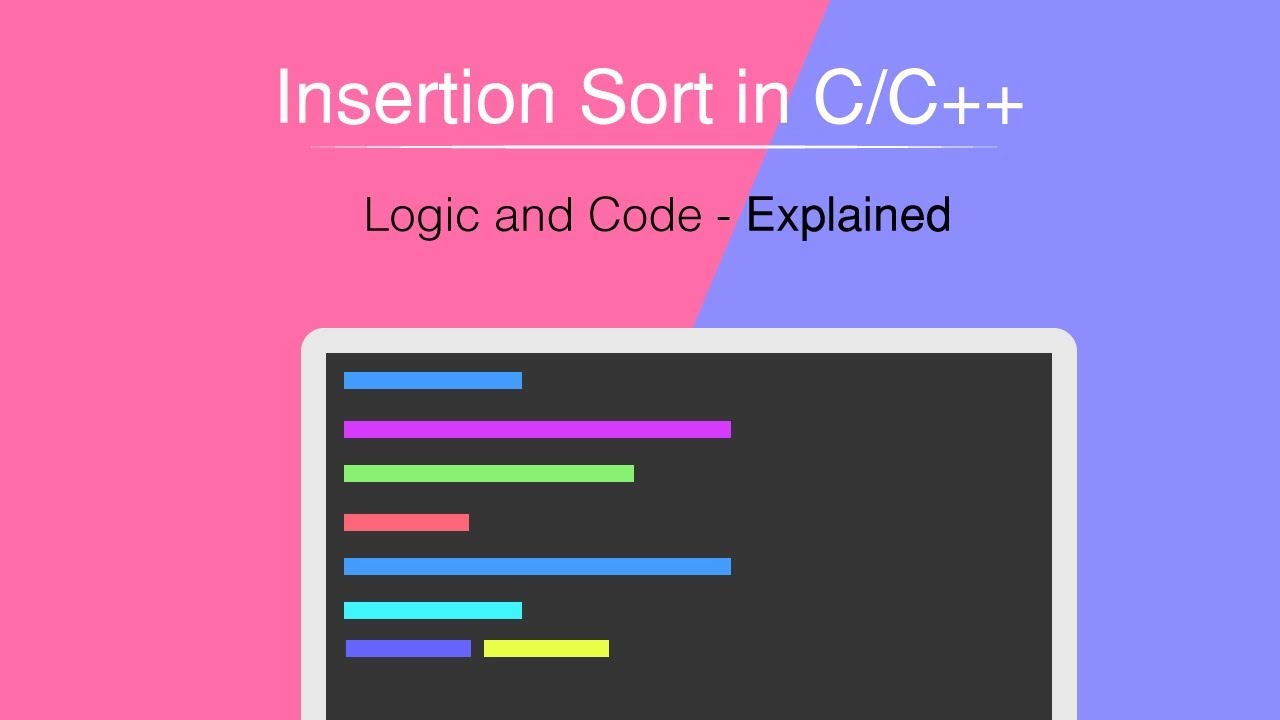
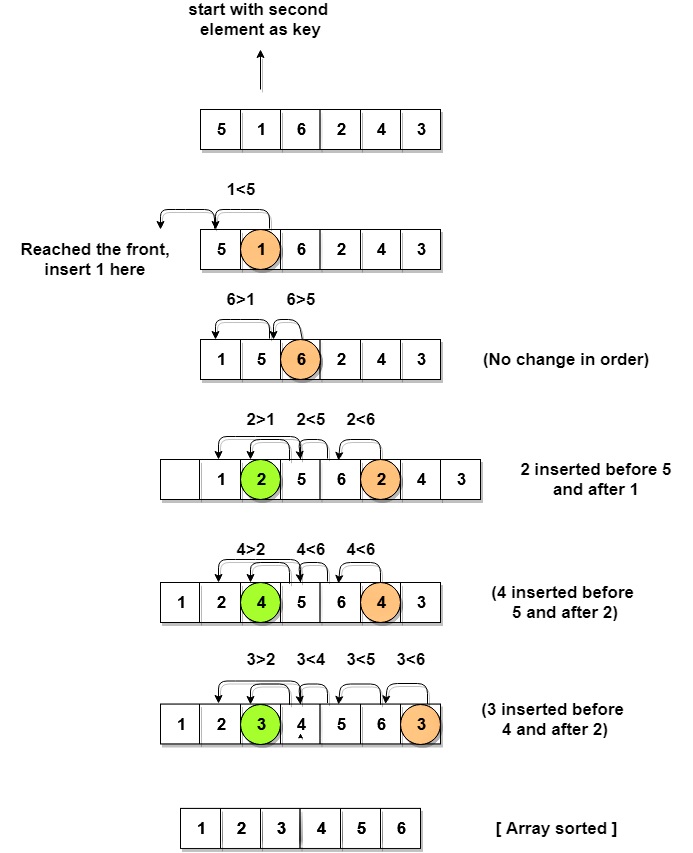
