Insertion sort is a sorting algorithm that places an unsorted element at its suitable place in each iteration. A few problems with the original code: Best case complexity of insertion sort is o (n), average and the worst case complexity is o (n 2).
Program to sort array using Insertion sort solution using
Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time.
89 17 8 12 0
#include <stdio.h> int main() { int n, array[1000], c, d, t; Note that c strings are null terminated, which simply means that a byte with the value of 0 signifies the end. Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time. To sort an array using insertion sort technique in c++ programming, you have to ask from user to enter the size for an array and then enter elements of that size in random order.
C program for sorting an array using bubble sort.
With a little modification, it will arrange numbers in descending order. Below is the source code for c program for sorting an array using insertion sort which is successfully compiled and run on windows system to produce desired output as shown below : 5 6 11 12 13. Therefore an array of strings is an array of arrays of chars (so your insertionsort signature is wrong).
Best case complexity of insertion sort is o (n), average and the worst case complexity is o (n 2 ).
C program for insertion sort to sort numbers. Insertion sort algorithm picks elements one by one and places it to the right position where it belongs in the sorted list of elements. Insertion sort | logical programming in c | by mr.srinivas** for online training registration: Jul 20, 2019 · insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands.
} } printf(sorted list in ascending order:\n);.
A) insert current node in sorted way in sorted or result list. 2) a string is an array of chars; I++) { scanf (%d, &a[i]); Insertion sort works similarly as we sort cards in our hand in a card game.
#include<stdio.h> int main() { int a[50], i,j,n,t;
Please refer complete article on insertion sort for more details! #include <stdio.h> int main () { int a [100], number, i, j, temp; Of elements in the list:\n); The question is, write a program in c++ to implement.
C++) { d = c;
Int size, arr [50], i, j, temp; 1) you cannot copy strings using =; Following c++ program ask to the user to enter array size and array element to sort the array using insertion sort technique, then display the sorted array on the screen: In the following c program we have implemented the same logic.
Some characteristics of insertion sort in c++.
0 enter 6 element :: 4 enter 2 element :: It’s more efficient with the partially sorted array or list, and worst with the descending order array and list. It is much less efficient on large lists than other algorithms such as quicksort, heapsort, or merge sort.
Following is the c program to sort the elements by using the insertion sort technique −.
#include<iostream.h> #include<conio.h> void main () { clrscr (); An array of data, and the total number in the array. This code implements insertion sort algorithm to arrange numbers of an array in ascending order. 1) create an empty sorted (or result) list 2) traverse the given list, do following for every node.
After receiving the inputs, sort the given array in ascending order using insertion sort as shown in the program given below:
Write a c program to sort a list of elements using the insertion sort algorithm. This code implements insertion sort algorithm to arrange numbers of an array in ascending order. 1 enter 3 element :: In this tutorial, you will learn about insertion sort algorithm and its implementation in c, c++, java and python.
With a little modification, it will arrange numbers in descending order.
Int n = sizeof(arr) / sizeof(arr [0]); This insertion sort in c program allows the user to enter the array size and the one dimensional array row elements. Before going through the program, lets see the steps of insertion sort with the help of an example. Use strncpy for that (using = only assigns pointers).
7 enter 5 element ::
3) change head of given linked list to. Int arr [] = { 12, 11, 13, 5, 6 }; C programming searching and sorting algorithm: 0 1 2 4 6 7 9 process returned 0
Printf (\n please enter the total number of elements :
6 enter 7 element :: Next, we are using nested for loop to sort the array elements using insertion sort. It sorts smaller arrays faster than any other sorting algorithm. } // insertion sort for (c = 1 ;
9 after insertion sort, sorted list is ::
C program for insertion sort using for loop. C program for insertion sort to sort numbers. Below is simple insertion sort algorithm for linked list. We assume that the first card is already sorted then, we select an unsorted card.
But, it is impractical to sort large arrays.
2 enter 4 element :: Insertion sort is the very simple and adaptive sorting techniques, widely used with small data items or data sets. Selection sort in c insertion sort in c. The insertion sort is useful for sorting a small set of data.
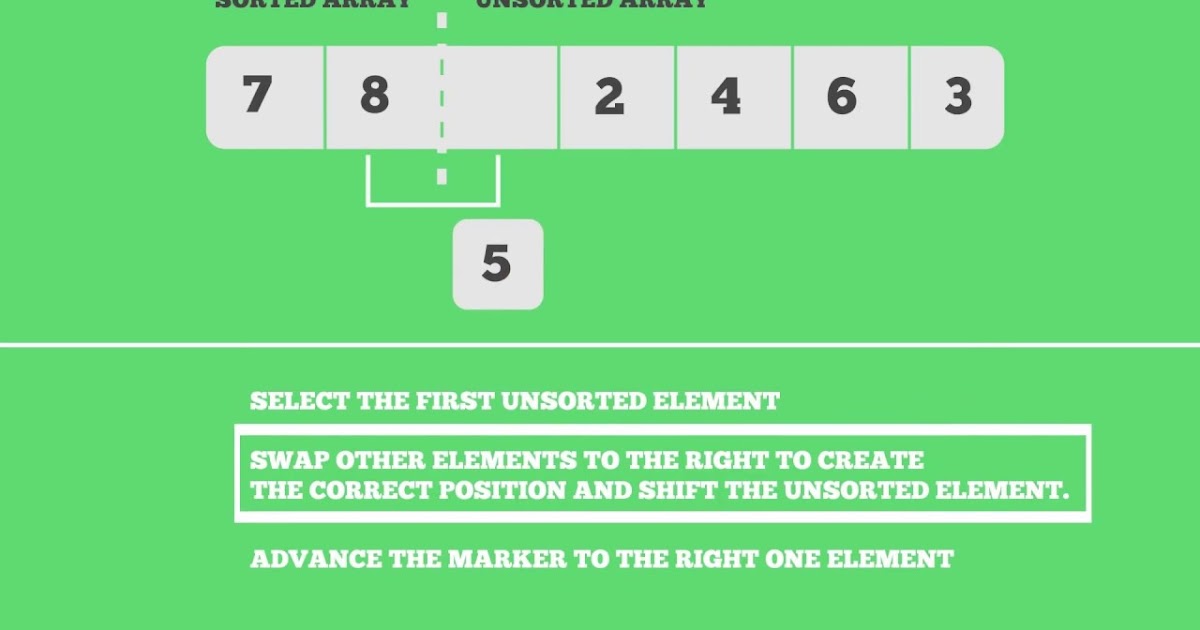
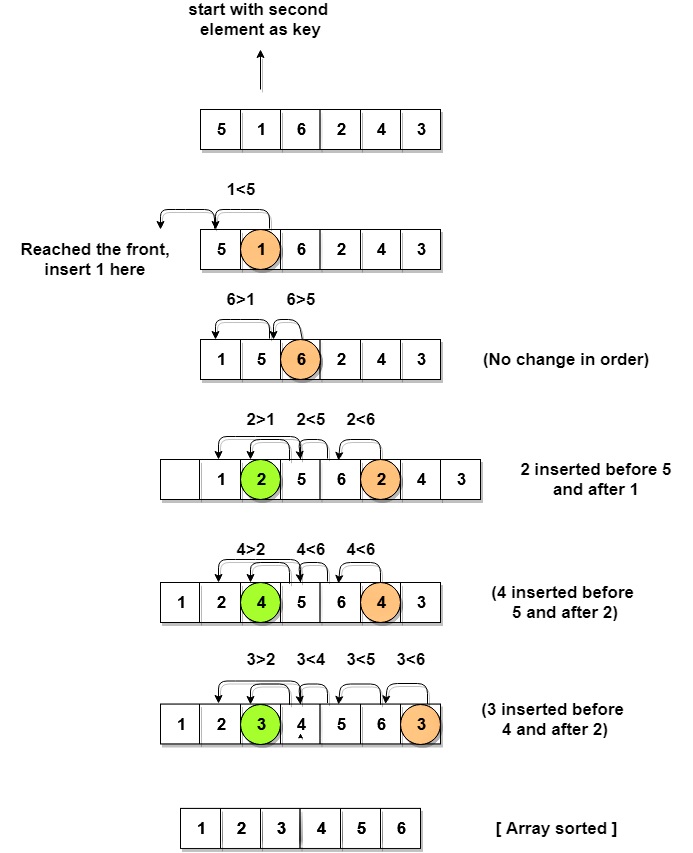

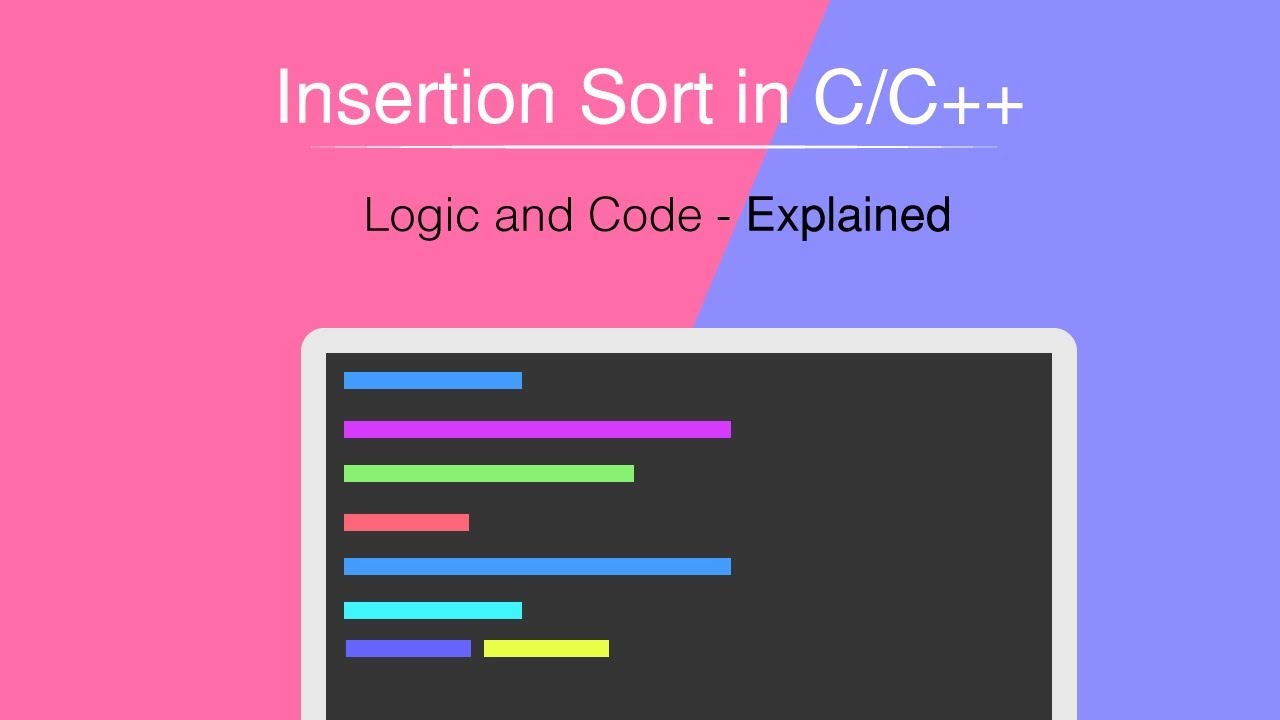


