In the below diagram, red color items are sorted and yellow ones are unsorted. Continue till the end of the array. I chose insertion sort and merge sort specifically.
Insertion sort algorithm in Java, Modula2, JavaScript and
While the term stable algorithm refers to the scenario where two equivalent elements appear identically, then a stable algorithm holds the elements at their relative positions after the execution of the.
Let insertionsort = (arr, n = arr.length) => { for(let i = 1;
Script to sort an array using the insertion sort algorithm function sort(arr) { //number of elements in the array var len = arr.length; Insertion sort in javascript implementation algorithm and pseudocode. Basically we are looking from left to right and sorting as we go. ++outer) as the algorithm goes through each element in order, it will:
In order to compare element by element, this insertion sort algorithm starts at the beginning of the datastore array and will continue to run until the end of the array has been reached.
.a) insert current node in sorted way in sorted or result list. Viewed 637 times 0 this question already has answers here: Console.log(insertionsort( [1, 8, 2, 4, 5])); Each iteration removes one element from the input data, finds the location it belongs within the sorted list, and inserts it there.
Write a javascript program to sort a list of elements using insertion sort.
It repeats until no input elements remain. 1) create an empty sorted (or result) list. It is much less efficient on large lists than more advanced algorithms such as quicksort, heapsort, or merge sort. Then we compare the unsorted item to see if it is larger than the previous element, if not we insert the new item.
Inputarr [j + 1] = inputarr [j];
Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time. Start at the second element (2). The last thing we are going to be looking at with insertion sort is the run time. Insertion sort is a simple sorting algorithm in which values from the unsorted part are picked and placed at the correct position in the sorted part.
Insertion sort is not the best algorithm than selection sort, bubble sort and merge sort in.
It repeats until no input elements remain. We start the for loop at 1 because in the first step, the. Insertion sort visualization using javascript. We have discussed insertion sort for arrays.
The element is then inserted in front of the smaller element.
A graphical example of insertion sort:. In this article we are going to discuss insertion sort for linked list. //sort in the ascending order while(j >= 0 && arr[j] > key) { arr[j + 1] = arr[j]; The insertion sort algorithm is a sorting algorithm that allows you to sort an array by inspecting the elements from left to right and placing larger elements to the right of the smaller elements.
} inputarr [j + 1] = key;
Insertion sort in javascript checking the loop invariant conditions. In the following instances, the insertion sort algorithm is used: The gif above shows how insertion sort works. It finds the correct position to insert the item in the sorted list that’s why it’s called insertion sort.
Below is a simple insertion sort algorithm for a linked list.
Insertion sort is a method of sorting an array by dividing the array into a 'sorted' portion and 'unsorted' portion. ● lastly, when there are few elements for sorting. Active 3 years, 11 months ago. Insertion sort algorithm in javascript [duplicate] ask question asked 3 years, 11 months ago.
● firstly, when only a few elements are in the list.
Insertion sort algorithm on javascript (4 answers) closed 3 years ago. At this point, you have made a series of inner passes and completed an outer pass. Insertion sort algorithm iterates, consuming one input element each repetition, and growing a sorted output list. It is a simple sorting algorithm used to arranging the array list in a sequence by using ascending or descending order.
Insertion sort maintains a sorted list and unsorted list in the same array.
This is accomplished by a for loop: Here, we are sharing the code f insertion sort in javascript implementation algorithm and pseudocode. For (var outer = 1; 2) traverse the given list, do following for every node.
At each iteration, insertion sort removes one element from the input data, finds the location it belongs within the sorted list, and inserts it there.
} arr[j + 1] = key; [1, 2, 4, 5, 8] In order to know more about it. Repeat the process until the entire array is sorted.
I'm writing the insertion sort algorithm in javascript.
Insertion sort is a simple and stable sorting algorithm that picks an element from an unsorted list and inserts it into the sorted list at the appropriate position. In order to know for sure that our algorithm is working correctly and not just. In this tutorial, we will learn about the insertion sort algorithm and its implementation in javascript. Pass the unsorted array [5, 2, 4, 6, 1, 3] into insertion sort.
The insertion sort algorithm starts by comparing your element at index 0 and index 1.when the element at index 1 is smaller than index 0, then the element at index 0 will be.
And that’s all there is to insertion sort! I++) { let key = arr[i];





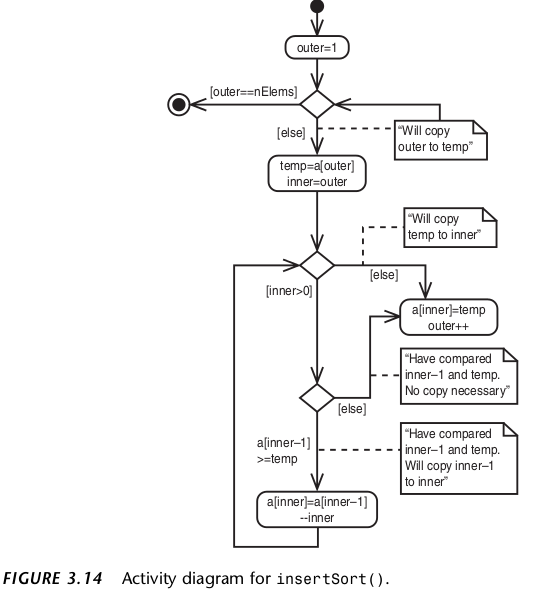
