It is much less efficient on large lists than more advanced algorithms such as quicksort, heapsort, or merge sort. The same approach is applied in insertion sort. The best case scenario would fetch run time complexity of o (n) when the data is already in sorted order.
C Sharp exercises Insertion sort w3resource
I++) { int current = arr[i];
Insertion sort program in c;
The array is virtually split into a sorted and an unsorted part. It has one of the simplest implementation it is efficient for smaller data sets, but very inefficient for larger lists. Place it in its corresponding position in the sorted part. Temporary = array [i] j = i.
Insertion sort is a sorting algorithm that places an unsorted element at its suitable place in each iteration.
This sorting algorithm sorts the array by shifting elements one by one. Insertion sort program in c using while loop; Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands. We assume that the first card is already sorted then, we select an unsorted card.
Step 1 − if the element is the first one, it is already sorted.
Int n = sizeof(arr) / sizeof(arr[0]); While (j >= 0 && arr[j] > current) { //swap arr[j + 1] = arr[j]; O (n*2) efficient for (quite) small data sets, much like other quadratic sorting algorithms. Explain the insertion sort by using c language.
Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time.
The insertion sort algorithm is performed using the following steps. Insertion sort is used to pick any. O (n*2) efficient for (quite) small data sets, much like other quadratic sorting. Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time.
Insertion sort is a simple sorting method for small data list, in this sorting technique one by one element shifted.
Insertion sort is less efficient than the other sorting. Insertion sort is one of the most common type of sorting method used in solving data structure problems. Insert the value if the correct position is found. Time complexity is o(n 2).
The “inner while loop” shifts the elements using swapping.
} } int main() { int arr[] = {13,46,24,52,20,9}; Space complexity is o (1). Aug 21, 2019 · algorithm for insertion sort. } arr[j + 1] = current;
In this tutorial, you will learn about insertion sort algorithm and its implementation in c, c++, java and python.
Shift the remaining elements accordingly. Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time. The concept used in insertion sort is further discussed in detail. Function insertion sort (data_type array [], integer variable n) begin.
Of elements in the list:\n);
Insertion sort algorithm is count in simple sorting algorithms. More efficient in practice than most other simple quadratic (i.e., o (n2)) algorithms such as selection sort or bubble sort. To sort an array of size n in ascending order: #include<stdio.h> void insertion_sort(int arr[], int n) { // insertion sort int i;
It is better than selection sort and bubble sort algorithms.
However, insertion sort provides several advantages like simple implementation, efficient for (quite) small data sets, more efficient in practice than. Compare the new picked element with the sorted element of an array. I++) { scanf (%d, &a[i]); Example of insertion sort with diagram;.
The idea behind the insertion sort is that first take one element, iterate it through the sorted array.
Jul 20, 2019 · insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands. Loop (i = 0, i < n, i++) begin. Declare integer variables i, j. Insertion sort is a stable sort.
Take an element from the unsorted array.
Following are some of the important characteristics of insertion sort. Insertion sort has very simple implementation and efficient for small data sets. Insertion sort is a simple sorting algorithm that works similar to the way you sort playing cards in your hands. In this approach, start iterating the “outer for loop” from the 2nd position of the array.
It builds the final sorted array by shifting one item at a time.
Now pick the new element which you want to insert. For (int i = 0; For example, insertion sort can be used in while playing card game where we can insert a card into a proper sequence in the stack of cards. The worst case is when the data is in reverse order which will be having a run time complexity of o (n2).
Take first element from the unsorted portion and insert that element into the sorted portion in the order specified.
Space complexity is o(1) because an extra variable key is used. If there is only one element or the first element in an array, it behaves as a sorted array. #include<stdio.h> int main() { int a[50], i,j,n,t; Following is the c program to sort the elements by using the insertion sort technique −.
Void insertionsort (int arr [], int n) {.
In the below dry run, Step 1 − if it is the first element, it is already sorted. Although it is simple to use, it is not appropriate for large data sets as the time complexity of insertion sort in the average case and worst case is o(n 2), where n is the number of items. Insertion sort works similarly as we sort cards in our hand in a card game.
Values from the unsorted part are picked and placed at the correct position in the sorted part.




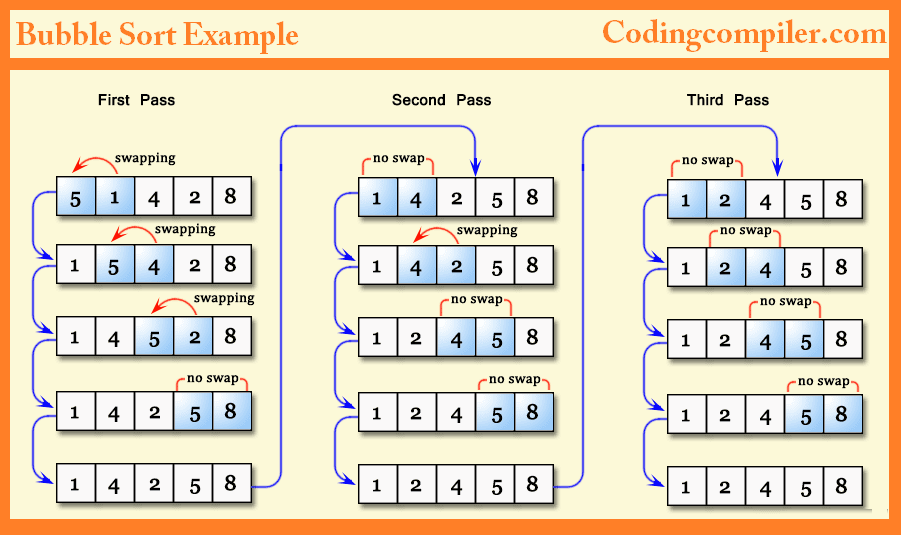
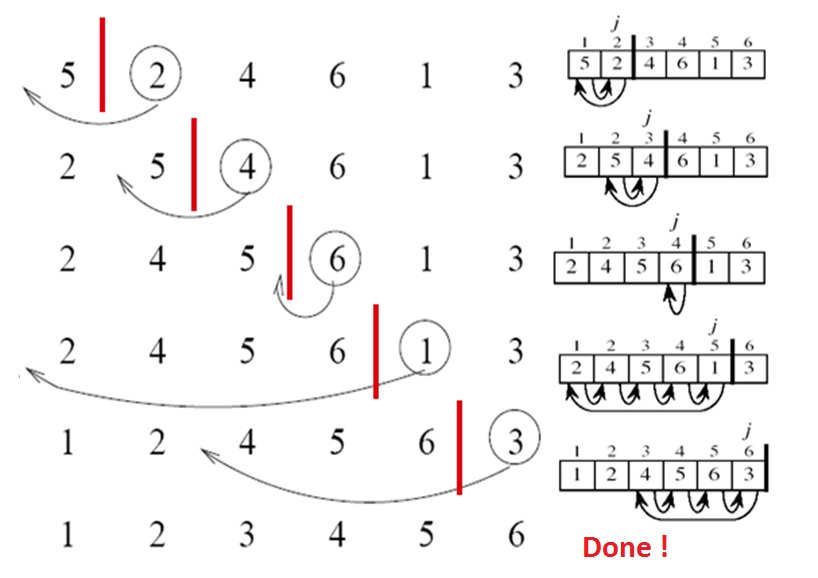
