Complexity analysis of the insertion sort algorithm. Below are the other sorting algorithm i have covered on my blog. Insertion sort is a sorting algorithm that helps in sorting objects of an array one by one.
Insertion Sort with Example and Time Complexity
Void insertionsort (int arr [], int n) {.
Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands.
I++) { scanf (%d, &a[i]); This article will demonstrate how to implement an insertion sort algorithm in c++. In insertion sort we pick first element and put it at its actual position by comparison with its right elements. Of elements in the list:\n);
The insertion sort iterates through each element in the.
While (j >= 0 && arr[j] > current) { //swap arr[j + 1] = arr[j]; Let x is an array of n elements. Hello friends, in this series to explain all the sorting algorithms in c#. With a little modification, it will arrange numbers in descending order.
Aug 21, 2019 · algorithm for insertion sort.
We assume that the first card is already sorted then, we select an unsorted card. This process will continue until array gets sorted. Step 1 − if the element is the first one, it is already sorted. An insertion sort is a sorting technique used in c programming to sort elements of an array in ascending or descending order.
Insertion sort works by picking one element at a time and places it accordingly in the array.
With each iteration, an element from the input is pick and inserts in the sorted list at the correct location. In insertion sort, input data is divided into two […] The insertion sorting c++ is implemented by the use of nested loops, it works in a way such that the key will be placed in. Int n = sizeof(arr) / sizeof(arr[0]);
In this guide, we will show you how to implement the insertion sort as a separate function that takes a reference to the std::vector object and modifies the contents in place.
Insertion sort is a sorting technique, which use to sort the data in ascending or descending order, like another sorting technique (selection, bubble, merge, heap, quicksort, radix, counting, bucket, shellsort, and comb sort). Best case complexity of insertion sort is o(n), average and the worst case complexity is o(n 2). Let x is an array of n elements. Implement the insertion sort for the std::vector container in c++.
C program for insertion sort to sort numbers.
X[0] may be considered as a sorted array of one element. Step by step explanation of insertion sort in swift. J=1 i.e unsorted position starts from 1. Step 1 − if it is the first element, it is already sorted.
Following is the c program to sort the elements by using the insertion sort technique −.
In this tutorial, you will learn about insertion sort algorithm and its implementation in c, c++, java and python. J=1 i.e unsorted position starts from 1. Explain the insertion sort by using c language. The insertion process begins from the second element since the first element can be directly placed in the sorted set at position 0.
Quick sort algorithm selection sort
Today i wish to discuss briefly about insertion sort, it’s. Step 3 − compare the current element with all elements in the sorted array. #include<stdio.h> void insertion_sort(int arr[], int n) { // insertion sort int i; This code implements insertion sort algorithm to arrange numbers of an array in ascending order.
} } int main() { int arr[] = {13,46,24,52,20,9};
Now we will pick each number from the unsorted section and insert that number at a. Insertion sort works similarly as we sort cards in our hand in a card game. And then select next element of an array and try to place it at its actual place after comparison. Insertion sort is a simple sorting algorithm.
In this sorting technique, we assume that the first number in the array is in the sorted section and the rest of all the other numbers in the array are in the unordered section.
This is my next article to have the best, easy and clear step by step explanation of insertion sort algorithm in c# with code example. #include<stdio.h> int main() { int a[50], i,j,n,t; Below is simple insertion sort algorithm for linked list. X[0] may be considered as a sorted array of one element.
It is preferred our selection sort but other faster algorithms like bubble sort, quicksort, and merge sort are preferred our insertion sort.
For (int i = 0; I++) { int current = arr[i]; Insertion sort is very easy and basic sorting technique. Another advantage of insertion sort is that it is a stable sort which means it maintains the order of equal elements in the list.
From the pseudo code and the illustration above, insertion sort is the efficient algorithm when compared to bubble sort or selection sort.
Insertion sort c++ is one of the most commonly used algorithm in c++ language for the sorting of fewer values or smaller arrays. It will keep working on single elements and eventually put them in the right position, eventually ending with a sorted array. Introduction to insertion sort in c. A) insert current node in sorted way in sorted or result list.
1) create an empty sorted (or result) list 2) traverse the given list, do following for every node.
It’s very useful with small data set or partially sorted data and not efficient if data is sorted in descending order and you want to sort data in. The insertion process begins from the second element since the first element can be directly placed in the sorted set at position 0. Insertion sort is a sorting algorithm that places an unsorted element at its suitable place in each iteration.



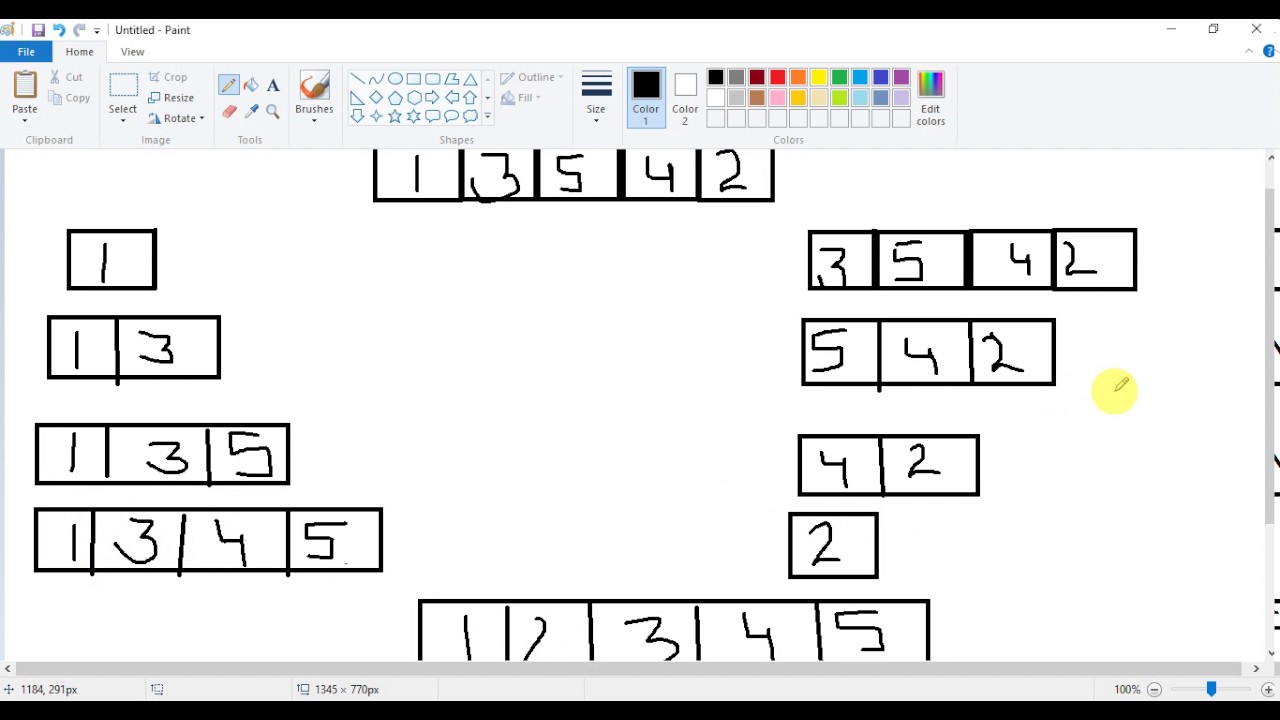
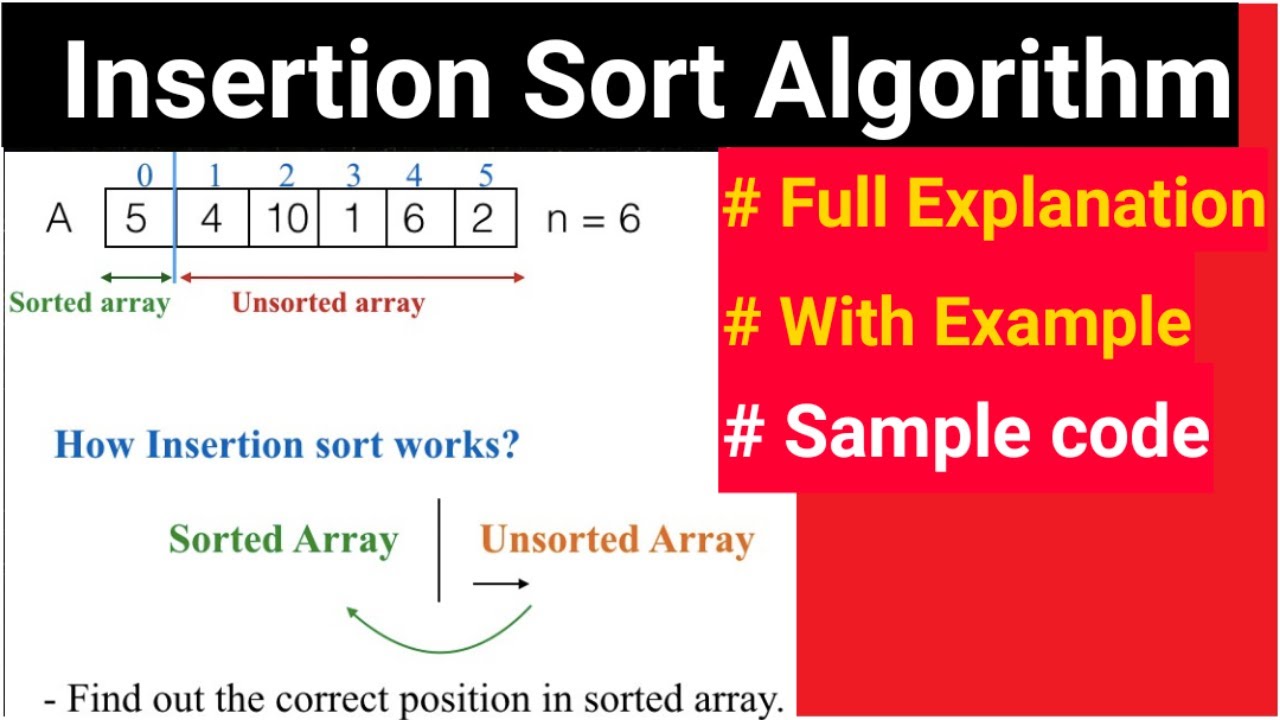

