#include<stdio.h> int main() { int a[50], i,j,n,t; Insertion sort is a simple sorting algorithm that works similar to the way you sort playing cards in your hands. Insertion sort algorithm and program in c.
Insertion Sort Program in C » PREP INSTA
Program for insertion sort in c.
Values from the unsorted part are picked and placed at the correct position in the sorted part.
Best case complexity of insertion sort is o (n), average and the worst case complexity is o (n 2 ). X [i+1]=key i.e insert key in correct position. Insertion sort is a sorting algorithm that places an unsorted element at its suitable place in each iteration. Iii) insertion sort algorithm and it’s implementation using c program.
Void insertionsort (int arr [], int n) {.
The insertion sort is useful for sorting a small set of data. J=j+1 i.e next unsorted element. C programming searching and sorting algorithm: Insertion sort algorithm picks elements one by one and places it to the right position where it belongs in the sorted list of elements.
Introduction to insertion sort in c.
I) what is insertion sort. [end of step 5 innerloop] 8. If j<n go to step 3. } arr[j + 1] = current;
Given an unsorted array, write a c program to sort an array using insertion sort.
We assume that the first card is already sorted then, we select an unsorted card. Insertion sort in c programming is the simple sorting algorithm. While (j >= 0 && arr[j] > current) { //swap arr[j + 1] = arr[j]; While x [i]>key and i>=0 then x [i+1]=x [i].
89 17 8 12 0
In this tutorial, we are going to discuss following points. About press copyright contact us creators advertise developers terms privacy policy & safety how youtube works test new features press copyright contact us creators. We select one element from the unsorted set at a time and insert it into its correct position in the sorted set. I++) { int current = arr[i];
For (int i = 0;
Int n = sizeof(arr) / sizeof(arr[0]); Of elements in the list:\n); #include<stdio.h> void insertion_sort(int arr[], int n) { // insertion sort int i; I.e shift element to the right.
} } int main() { int arr[] = {13,46,24,52,20,9};
Aug 21, 2019 · algorithm for insertion sort. Algorithm to sort an array of size n in ascending order: In this tutorial, you will learn about insertion sort algorithm and its implementation in c, c++, java and python. This code implements insertion sort algorithm to arrange numbers of an array in ascending order.
Insertion sort is a sorting algorithm that helps in sorting objects of an array one by one.
Set arr[j+1]=temp [insert element in proper place] [end of step 2 outerloop] 9. Insertion sort works similarly as we sort cards in our hand in a card game. The basic idea of this method is to place an unsorted element into its correct position in a growing sorted list of elements. Explain the insertion sort by using c language.
Step 3 − compare the current element with all elements in the sorted array.
C program for insertion sort to sort numbers. Insertion sort program in c. Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time. Write a c program to sort a list of elements using the insertion sort algorithm.
In the following c program we have implemented the same logic.
Step 1 − if the element is the first one, it is already sorted. An insertion sort is a sorting technique used in c programming to sort elements of an array in ascending or descending order. The best case scenario would fetch run time complexity of o(n) when the data is already in sorted order. Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands.
It will keep working on single elements and eventually put them in the right position, eventually ending with a sorted array.
It is much less efficient on large lists than other algorithms such as quicksort. In this sorting technique, we assume that the first number in the array is in the sorted section and the rest of all the other numbers in the array are in the unordered section. The worst case is when the data is in reverse order which will be having a run time complexity of. I++) { scanf (%d, &a[i]);
As the name suggests, this algorithm just compares two elements in the array and insert it in the appropriate place.
Ii) how it’s different from selection sort and bubble sort. It will insert a new element when found the. Insertion sort works by picking one element at a time and places it accordingly in the array. Following is the c program to sort the elements by using the insertion sort technique −.
Before going through the program, lets see the steps of insertion sort with the help of an example.
Printf(enter the number of elements:); The insertion_sorted() function takes an array as input and applies insertion sort algorithm on that. With a little modification, it will arrange numbers in descending order. But, it is impractical to sort large arrays.
Now we will pick each number from the unsorted section and insert that number at a.
The array is virtually split into a sorted and an unsorted part.





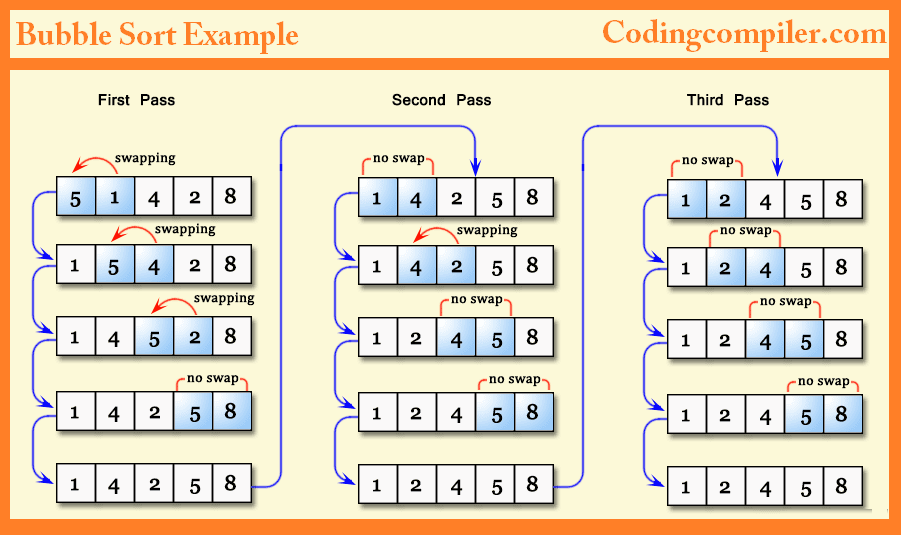
