Step 1 − create a new node at the end of heap. Step 2 − assign new value to the node. In the heap sort algorithm, we insert all the elements from the unsorted list or array into a heap.
Heap sort algorithm program in C and C++ Edusera
Efficient sorting is important for optimizing the efficiency of other algorithms (such as search and merge algorithms) that require input data to be in sorted lists.
Begin if heap is full, then exit else n := n + 1 for i := n, i > 1, set i := i / 2 in each iteration, do if item <= heap [i/2], then break heap [i] = heap [i/2] done end if heap [i] := item end.
17 — describe the heap sort algorithm use the buildmaxheap () method on the list. Reduce the considered range of the list by one. Taking into account completeness of the tree, o(h) = o(log n), where n is number of elements in a heap. We can optimize insertion sort further using binary search.
Insertion sort is a stable sorting algorithm.
Insertion sort is one of the fastest sorting algorithms for the partially or almost sorted input data, i.e., the time complexity is o(kn), where each element is no more than k places away from its sorted position. Public void insert(int value) { if (heapsize == data. Reconstruct the heap by placing or interchanging the root with the last element. The heap size will now shrink by 1.
This process is continued until there is only one.
Heap sort reconstructs the heap after each extraction. Worst case = average case = best case = o(n log n) Here’s the algorithm for heap sort: Heap sort algorithm for sorting in increasing order:
New root may violate max heap property, but its children are max heaps.
10 / \ 5 3 / \ 2 4 the new element to be inserted is 15. At any point of time, heap must maintain its property. Build a max heap from the input data. 10 / \ 5 3 / \ / 2 4 15 step 2 :
The heap is updated after.
Repeat step 2 while the size of the heap is greater than 1. Insert the new element at the end. Firstly choose root as an elevated element from the given information set of elements to create a maximal heap. At this point, the largest item is stored at the root of the heap.
The heap sort algorithm is simpler to understand than other equally efficient sorting algorithms, because it does not use recursion.
Heap sort takes linear time to sort an array of n elements. Creating a heap of the unsorted list/array. A[i], a[0] = a[0], a[i] heap_adjust(a, 0, i) or Heap sort is a stable sorting algorithm.
Then, we heapify the first element.
Build a max heap to sort in increasing order, build a min heap to sort in decreasing order. Algorithm of heap sort in c++. There are two classes of sorting algorithms: In heap sort, we convert the array into a heap.
Swapping the root element with the last element of the heap (rightmost leaf node) and reducing heap size by 1.
Build max heap from unordered array; Finally, heapify the root of the tree. Build a heap from the input data. Then a sorted array is created by repeatedly removing the largest/smallest element from the heap, and inserting it into the array.
In o (n) operations, this function, also known as heapify (), constructs a.
Heap sort code examples def heap_sort(a): Heap sort algorithm inserts all elements (from an unsorted array) into a heap then swap the first element (maximum) with the last element (minimum) and reduce the heap size (array size) by one because last element is already at its final location in the sorted array. Now max element is at the end of the array! Use the siftdown () method on the.
The heapsort algorithm has two main parts (that will be broken down further below):
Complexity of the insertion operation is o(h), where h is heap's height. Change the first and last components of the list’s order. Replace it with the last item of the heap followed by reducing the size of heap by 1. In computer science, a sorting algorithm is an algorithm that puts elements of a list into an order.the most frequently used orders are numerical order and lexicographical order, and either ascending or descending.efficient sorting is important for optimizing the efficiency of other algorithms (such as search and merge algorithms) that require input data to be in sorted lists.
While insertion, we also assume that we are inserting a node in an already heapified tree.
It will keep working on single elements and eventually put them in the right position, eventually ending with a. Run max_heapify to fix this. Length) throw new heapexception(heap's underlying storage is overflow); Heap sort algorithm is divided into two basic parts:
Insertion sort is a sorting algorithm that helps in sorting objects of an array one by one.
Insertion sort works by picking one element at a time and places it accordingly in the array. Then we keep extracting the maximum element from the heap and place it accordingly. Request pdf | on may 5, 2021, inayatullah soomro and others published performance analysis of heap sort and insertion sort algorithm | find, read and cite all. Swap elements a[n] and a[1] :
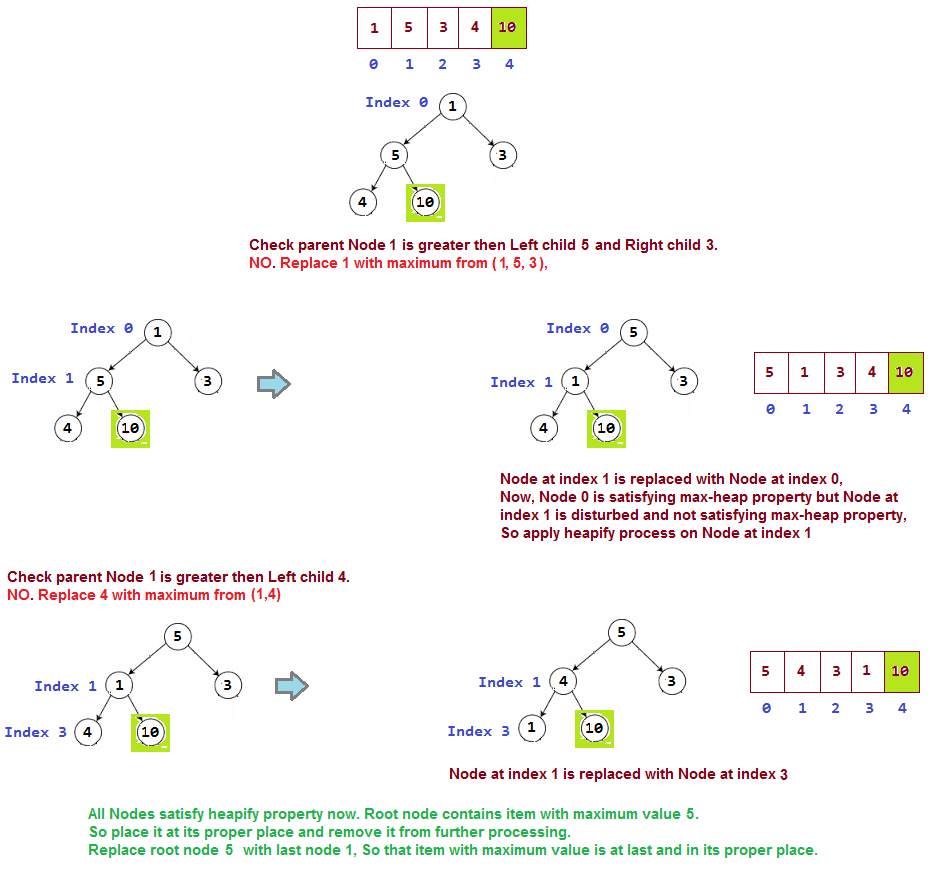





